Adhaar Card Validation
-
To validate Aadhar Card number, we adopt Verhoeff algorithm.
-
UIDAI (Unique Identification Authority of India) uses Verhoeff algorithm and it handles various data entry errors.
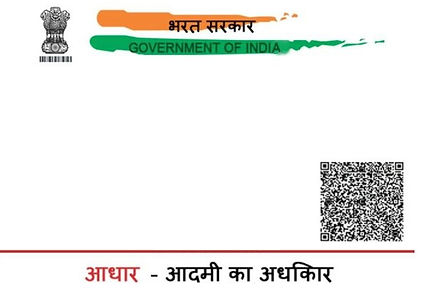
7564 6239 1883
Birendra Pradhan
Father: Babu Pradhan
DOB: 22/07/1995
MALE
Aadhar generated number
Check Digits (Generated by Verhoeff algorithm)
VERHOEFF ALGORITHM
-
It is a checksum algorithm.
-
In 1969, a Dutch mathematician named Verhoeff carried out a study of errors made by humans in handling decimal numbers.
-
It also works for generating and validating the checksum digits of :
-
Credit Card Validation
-
Debit Card
-
ISBN
-
Account Number
-
Most errors happens:
-
Single errors: a changed to b (60 to 95 percent of all errors)
-
Adjacent Transpositions: ab changed to ba(10 to 20 percent)
-
Twin errors: aa changed to bb (0.5 to 1.5 percent)
-
Jump transpositions: acb changed to bca (0.5 to 1.5 percent)
-
Omitting or adding a digit: (10 to 20 percent)
HOW IT WORKS
The Verhoeff algorithm can be implemented using three tables:
Multiplication table d
-
The first table, d, is based on multiplication in the dihedral group D5.
-
Note that this group is not commutative, that is, for some values of j and k, d(j,k) ≠ d(k, j).
Inverse table inv
-
The inverse table inv represents the multiplicative inverse of a digit, that is, the value that satisfies d(j, inv(j)) = 0.
Permutation table p
-
The permutation table p applies a permutation to each digit based on its position in the number.
Multiplication table d
Permutation table p
Inverse table inv
-
Inversion table required for getting checksum.
-
Here is an example of validating a given number using Verhoeff Algorithm.
Validate the check digit 2363:
-
Put index number in i according to Aadhar no.
-
Put your Aadhar number in ni column on the table, taken from right to left.
-
Initialize the checksum c to zero.
-
For each index, i of the array n, starting at zero, replace c with d(c, p(i mod 8, ni)).
-
If c is zero, so the check is correct.
i = Index number
ni = Given number
(Right to left)
Given
Calculate
(From above table)
c = 0
The number is Valid.
STRENGTH
-
it can detect more transcription errors than Luhn.
-
It is special as it has the property of detecting 95.3% of the phonetic errors.
-
The strengths of the algorithm are that it detects :
-
All transliteration and transposition errors
-
Additionally most twin
-
Twin jump
-
Jump transposition
WEAKNESS
-
The main weakness of the Verhoeff algorithm is its complexity
-
Calculations required cannot readily be performed by hand.
VERHOEFF ALGORITHM IMPLEMENTATION
-
Here is the JAVA program of Verhoeff algorithm, used for creating the checksum or validate the checksum digit.
-
Used in client side to validate:
-
Aadhar Card Number
-
Credit Card
-
Debit Card
-
ISBN (International Standard Book Number)
-
and more
JAVA PROGRAM for validation of Aadhar card
import java.util.*;
public class Verhoeff {
public static void main(String [] args){
Scanner sc=new Scanner(System.in);
System.out.println("Enter your Aadhar Card no :");
String s=sc.nextLine();
System.out.println("Entered card is = " + validateVerhoeff(s));
}
// The multiplication table
static int [ ] [ ] d = new int [ ][ ]
{
{0, 1, 2, 3, 4, 5, 6, 7, 8, 9},
{1, 2, 3, 4, 0, 6, 7, 8, 9, 5},
{2, 3, 4, 0, 1, 7, 8, 9, 5, 6},
{3, 4, 0, 1, 2, 8, 9, 5, 6, 7},
{4, 0, 1, 2, 3, 9, 5, 6, 7, 8},
{5, 9, 8, 7, 6, 0, 4, 3, 2, 1},
{6, 5, 9, 8, 7, 1, 0, 4, 3, 2},
{7, 6, 5, 9, 8, 2, 1, 0, 4, 3},
{8, 7, 6, 5, 9, 3, 2, 1, 0, 4},
{9, 8, 7, 6, 5, 4, 3, 2, 1, 0}
};
// The permutation table
static int [ ] [ ] p = new int [ ] [ ]
{
{0, 1, 2, 3, 4, 5, 6, 7, 8, 9},
{1, 5, 7, 6, 2, 8, 3, 0, 9, 4},
{5, 8, 0, 3, 7, 9, 6, 1, 4, 2},
{8, 9, 1, 6, 0, 4, 3, 5, 2, 7},
{9, 4, 5, 3, 1, 2, 6, 8, 7, 0},
{4, 2, 8, 6, 5, 7, 3, 9, 0, 1},
{2, 7, 9, 3, 8, 0, 6, 4, 1, 5},
{7, 0, 4, 6, 9, 1, 3, 2, 5, 8}
};
// The inverse table
static int [ ] inv = {0, 4, 3, 2, 1, 5, 6, 7, 8, 9};
/* * For a given number generates a Verhoeff digit * */
public static String generateVerhoeff(String num){
int c = 0;
int [ ] myArray = stringToReversedIntArray(num);
for(int i = 0; i < myArray.length; i++)
{
c = d [c] [p [ ( ( i + 1) % 8)] [myArray [i]] ];
}
return Integer.toString( inv [ c ] );
}
/* * Validates that an entered number is Verhoeff compliant. * NB: Make sure the check digit is the last one. */
public static boolean validateVerhoeff(String num){
int c = 0; int [ ] myArray = stringToReversedIntArray(num);
for (int i = 0 ; i < myArray.length ; i++) {
c = d [c] [p[(i % 8)] [myArray[i] ] ];
}
return ( c == 0 );
}
/* * Converts a string to a reversed integer array. */
private static int [ ] stringToReversedIntArray(String num){
int [ ] myArray = new int[num.length()];
for (int i = 0; i < num.length(); i++) {
myArray [ i ] = Integer.parseInt(num.substring(i, i + 1));
}
myArray = reverse(myArray);
return myArray;
}
/* * Reverses an int array */
private static int [ ] reverse(int[] myArray) {
int [ ] reversed = new int[myArray.length];
for ( int i = 0; i < myArray.length ; i++ ) {
reversed [ i ] = myArray[myArray.length - (i + 1)];
}
return reversed;
}
}
-
Screenshot of the Aadhar Card validation program.
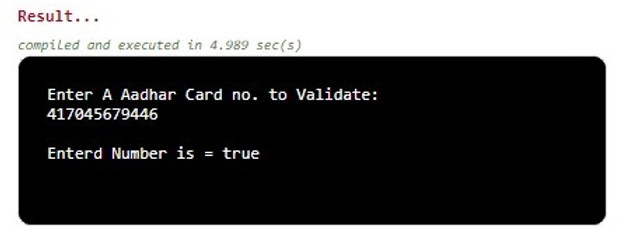