top of page
Java
What is it?
-
Java is a programming language.
-
A computing platform for application development.
-
It is one of the most used programming languages.
-
Java runs on a variety of platforms, such as Windows, Mac OS, and the various versions of UNIX.

History
-
James Gosling initiated Java language project in June 1991.
-
It was first released by Sun Microsystem in 1995.
-
Later acquired by Oracle Corporation.
Developed by Sun Microsystem
in 1995
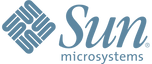
Acquired by Oracle
in 2010

Why Java
Platform Independent
-
Unlike C, C++, when Java is compiled, it is not compiled into platform specific machine, rather into platform-independent bytecode.
-
Bytecode is distributed over the web and interpreted by the JVM.
Object Oriented:
-
In Java, everything is an Object.
Secure
-
It uses public-key encryption to provide virus-free, tamper-free system.
Multithreaded:
-
Possible to write programs that can perform many tasks simultaneously.
-
This design feature allows the developers to construct interactive applications.
High Perpormance
-
With the use of Just-In-Time compiler, java enables high performance.
Environment Setup
-
JDK - Java development Kit allows us to code and runs Java programs.
-
JRE - Java Runtime Environment consists of the Java Virtual Machine (JVM), core classes, and supporting files. It provides the minimum requirements for executing a Java application.
-
JVM - Java Virtual Machine is an interpreter responsible for executing the java program line by line.
-
Here are some steps, how to set up Java on our computer.
Step 1: Download JDK
-
Go to link.
-
Accept T&C, click on Download JDK.
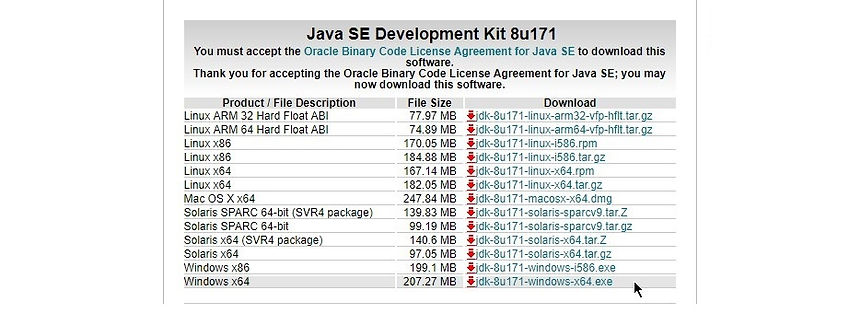
-
Once download, open the execution file and install JDK.

Step 2: Setting up the Path
-
To work with Java, we need to specify the PATH where the Java executable files are.
-
Pathe variable gives the location of executable files like javac, java etc; C:\Program Files\Java\jdk1.8.0_131\bin\.
-
Right-click on 'My Computer' > 'Properties' > 'Advanced' tab > click the 'Environment variables'.
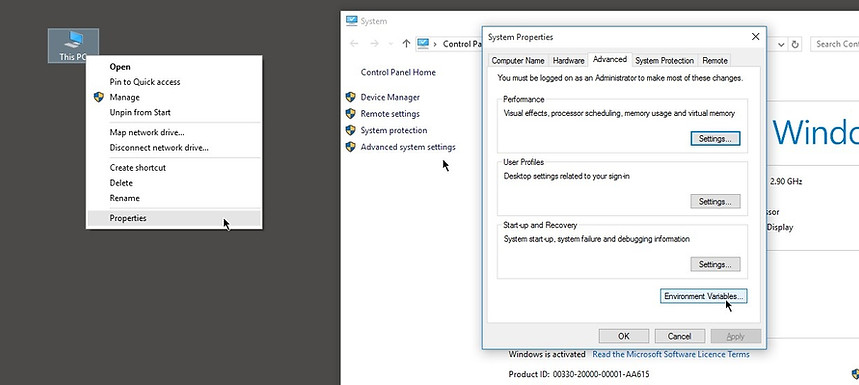
-
Click on new Button of User variables if no Path variable is there.
-
Copy the path of Java bin folder > set variable name as "PATH" > set variable value as path "C:\Program Files\Java\jdk1.8.0_171\bin" > click OK.

Paste the Java bin path
Step 3: Setting up the Path
-
You can follow a similar process to set CLASSPATH.
-
Click New > set variable name "Classpath" > set variable value as "C:\Program Files\Java\jdk1.8.0_171\lib"
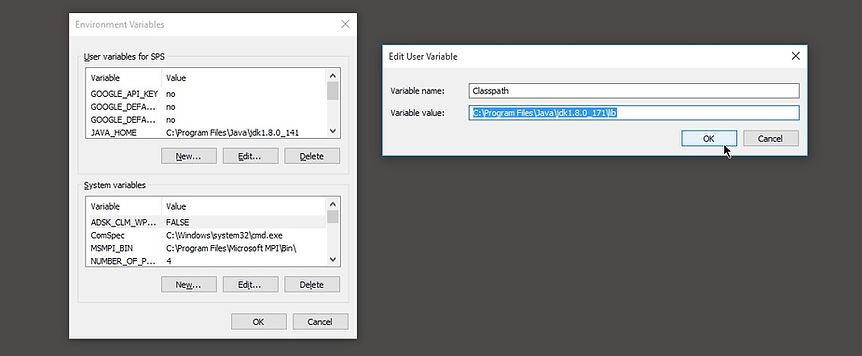
Step 4: Check the java command
-
To check whether Java is set-up or not, run any java commands in command line.
-
Here we will check the Java version as following.
-
Open Command Prompt > type "java -version" > click Enter.
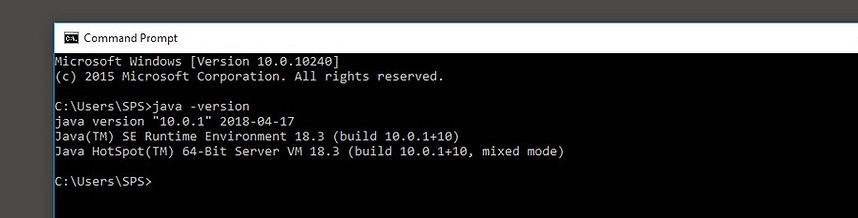
Checking of Java set-up
OOPs Concept
-
Java is an Object-Oriented Language.
-
It allows users to create the objects that they want and then create methods to handle those objects.
Class
-
It is only a logical component and not the physical entity.
-
It contains variable & properties.
Object
-
It is the instance of an Object.
-
There can be multiple instances of a single class.
Abstraction
-
Representing essential features without including background details.
Encapsulation
-
The process wrapping the data and methods together as a single unit.
-
The variables of a class will be hidden from other classes, also known as data hiding.
Inheritance
-
The process where one class acquires the properties (methods and fields) of another.
-
A inherits B, means class A acquires the properties of class B.
Polymorphism
-
The ability of an a variable, object or function to take on many forms.
Java Syntax
-
Unlike C, C++, when Java is compiled, it is not compiled into platform specific machine, rather into platform-independent bytecode.
-
Bytecode is distributed over the web and interpreted by the JVM.
Datatypes
-
A variable provides us with named storage.
-
Each variable in Java has a specific type.
Datatypes
Premitive
Non-Premitive
Boolean
Numeric
Character
Integral
Integer
Floating-point
boolean
char
byte
short
int
long
float
double
String
Array
etc
-
Here is a table have datatype syntax with default size and description.
Modifier
-
There are 2 types of modifier in Java.
-
Access Modifier:
-
default - Visible to the package.
-
private - Visible to the class only.
-
public - Visible to all package & classes.
-
protected - Visible to the package and all subclasses.
-
Non-Access Modifier:
-
static - For creating class methods and variables.
-
final - For finalizing the implementation of class, method, and variables.
-
abstract - For creating abstract classes and methods.
-
synchronized - Used for the method as threads.
-
volatile - Used for the variable in threading concept.
Declaring Variable
-
Declaring variable means set a data type to a variable.
-
Then we can initialize with a data.
-
Local variables are declared in methods, constructors, or blocks.
-
Instance variables are declared in a class, but outside a method, constructor or any block.
-
Class variables also known as static variables are declared with the static keyword

Datatype
Name
Hello World
-
To run java program we need JDK and a text editor.
-
Here we used Notepad++ to write "The Hello World" program.
-
Save as HelloWorld.java
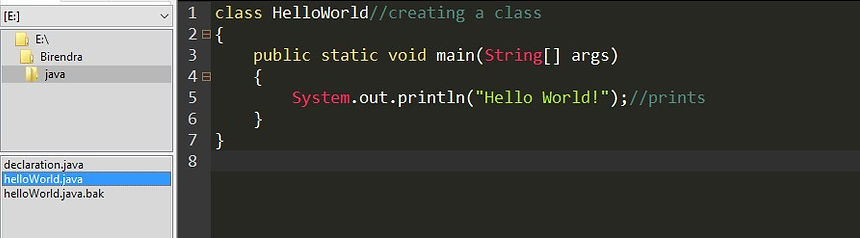
-
To compile the code, open Command prompt.
-
Go the directory > type javac HelloWorld.java
-
To execute, type java HelloWorld
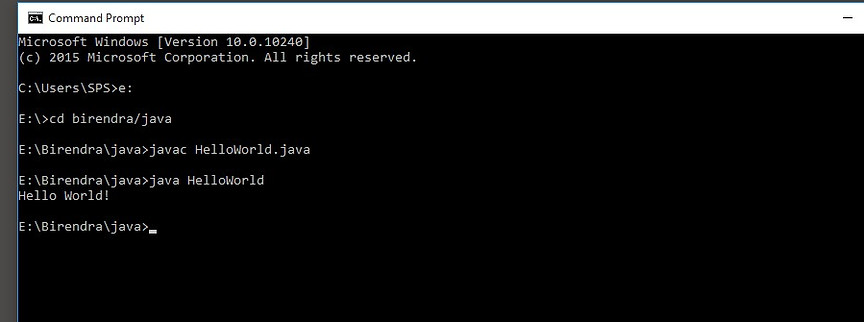
Go to directory
Compile the .java file
Execute the .class file
Here the ouput is.
Java Array
-
An array is an object contains a collection of similar type of elements.
-
It has a contiguous memory location
-
It is index-based, the first element of the array is stored at 0 indexes.
-
Here is the declaration, instantiation & initialization of the 1-d array.
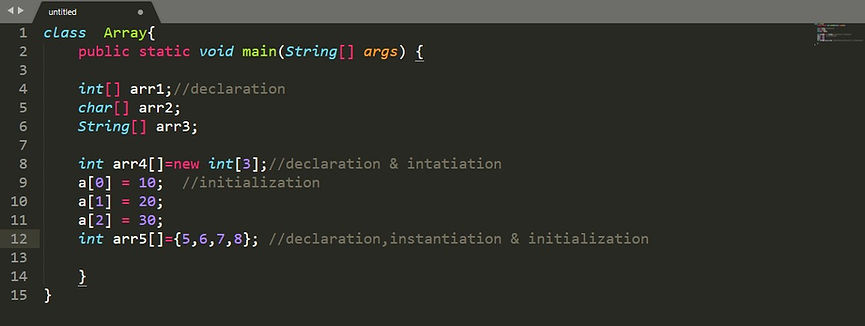
-
Multidimensional java array has rows and columns.
-
It can have 2-d or 3-d array.
-
Here is an example of multidimensional Array in java.
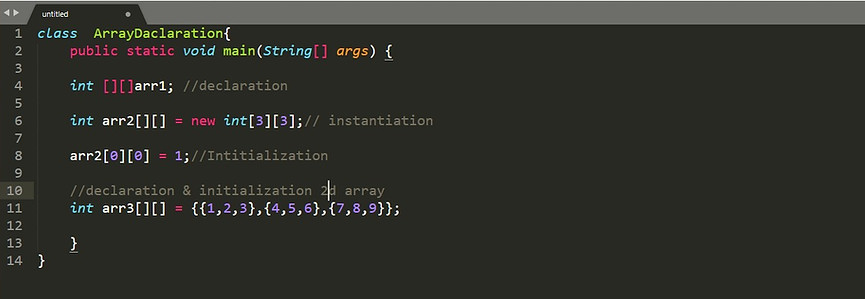
Class
-
The class is a template or blueprint from which objects are created.
-
It is a logical entity.
-
It can have:
-
fields
-
methods
-
constructors
-
blocks
-
nested class and interface
Object
-
The object is an instance of a class.
-
The new keyword is used to allocate memory at runtime.
-
All object gets memory in Heap area.
Method
-
In Java, a method is like function.
-
It improves code reusability.
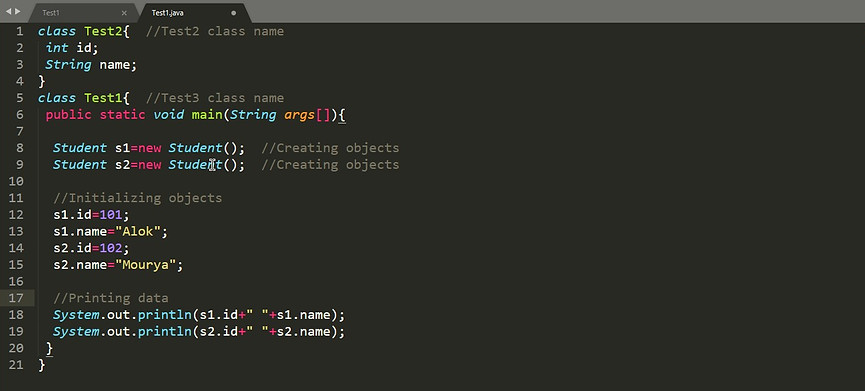
Constructor
-
In Java, constructor is a block of codes similar to method.
-
It is called when an instance of object is created.
-
There are 2 rules for creating java constructor.
-
Constructor name must be same as its class name.
-
Must have no explicit return type.
-
There are 2-types of constructor.
-
Default constructor
-
Parameterized constructor
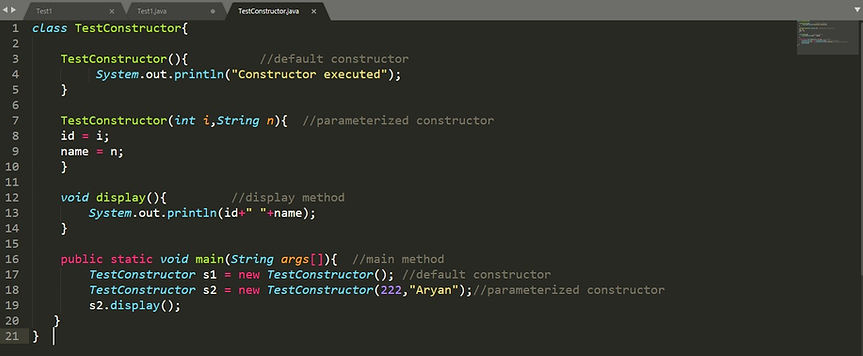
Collection Framework
Datastructures
-
Data structure provided by the Java utility package.
-
It has a wide range of functions to handle data.
-
Java introduced a new framework called Collection Framework
Collection Interface
-
Java provided ad hoc classes such as Dictionary, Vector, Stack, and Properties to store and manipulate groups of objects.
-
The java.util package contains all the classes and interfaces for Collection framework.
-
Here the Collection hierarchy of the Class and Interfaces.
HashSet
Unordered, allow Null, Not Sync
Set
LinkedSet
Not Sync, Ordered (maintains doubly linked )
No Duplicate
SortedSet
TreeSet
Not sync, Guarantees natural order,faster
ArrayList
Not Syncronized
Collection
List
Vector
Stack
Ordered Collection
Synchronized
LinkedList
Not Synchronized, work as list, stack,queue
Queue
ArrayDeque
Null allows
Multiple elements prior to process
PriorityQueue
No Null, Order on basis of priority heap
Interface
Class
Control Statement
-
With control statement, we can control the order of execution of the program, based on logic and values.
-
In Java the control statement divided into following categories.
Control Statement
Selection Statement
if
if-else
switch
Iteration Statement
while
do
for
Jump Statement
break
continue
return
-
With control statement, we can control the order of execution of the program, based on logic and values.
-
In Java the control statement divided into following categories.
if-else
-
Used to test the condition.
-
It checks boolean condition: true or false.
-
There are various type of if statement in Java:
-
if
-
if-else
-
if-else if ladder
-
nested if

switch
-
Executes one statement from multiple conditions.
-
It executed top to bottom once match the condition if no break statement (not default case).
-
We can write integer or string value in the case statement.
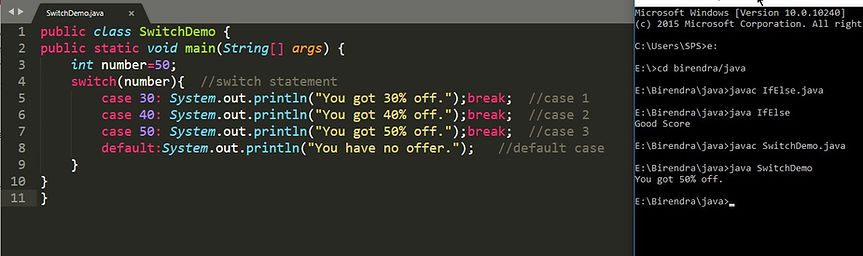
for loop
-
Loops are used to execute a set of instructions/functions repeatedly when some conditions become true.
-
For loop is used to iterate a part of the program several times.
-
If a number of iteration is fixed, for loop is recommended.
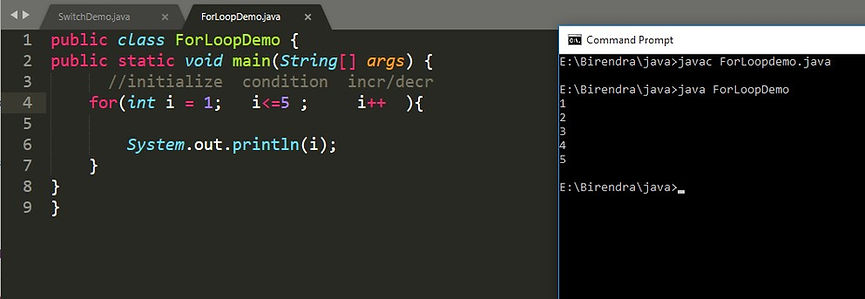
for-each loop
-
Used to traverse array or collection in java.
-
It does not work with increment/decrement.
-
It iterates the data based on the index value of an array.
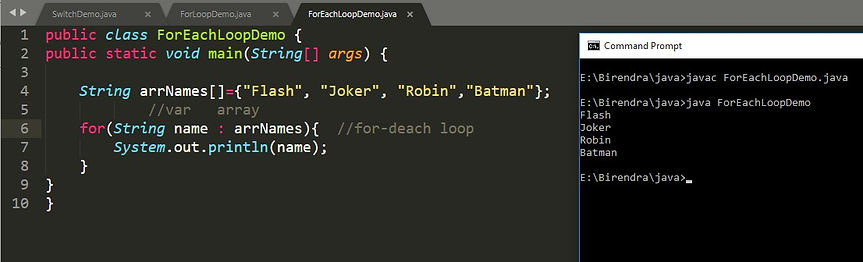
-
Labeled for loop is used a loop inside a for loop.
-
It is useful if we have nested for loop so that we can break/continue specific for a loop.
while
-
It is used to iterate a part of the program several times.
-
It is used if the number of iteration is not fixed.
-
If we pass true in condition, it will go infinitive loop.
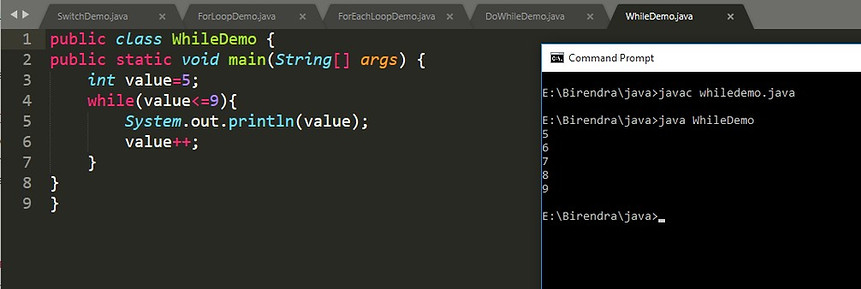
do while
-
It is executed at least once because the condition is checked after loop body.
-
It will have infinity loop, it the condition is TRUE.
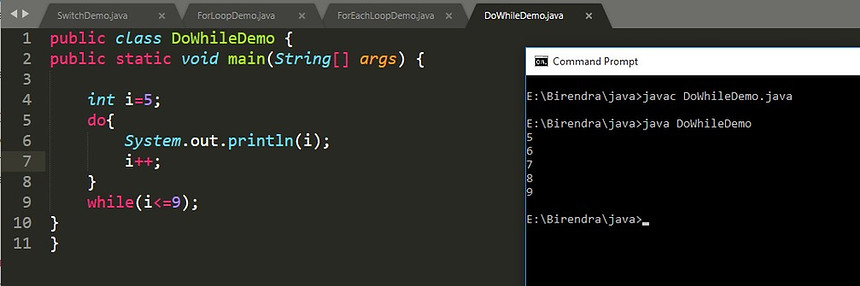
break & continue
-
In case of the inner loop, break breaks the only inner loop.
-
Continue is used to test the condition.
-
It checks boolean condition: true or false.
-
There are various type of if statement in Java:
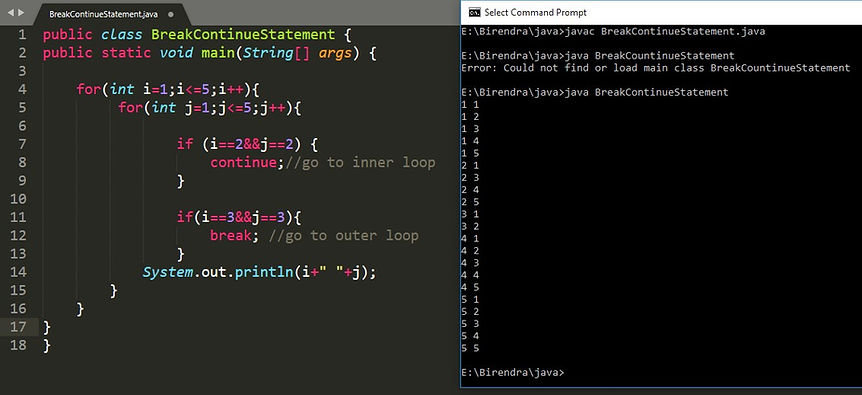
(2==2), inner loop continue
(3==3), inner loop broke
String in Java
-
It is a sequence of characters.
-
In Java programming language, strings are treated as objects.
-
In java String class allows to create and manipulate strings.
String Class
-
The java.lang.String class implements Serializable, Comparable and CharSequenceinterfaces.
-
There are two ways to create string in Java:
-
String literal
-
Using new keyword
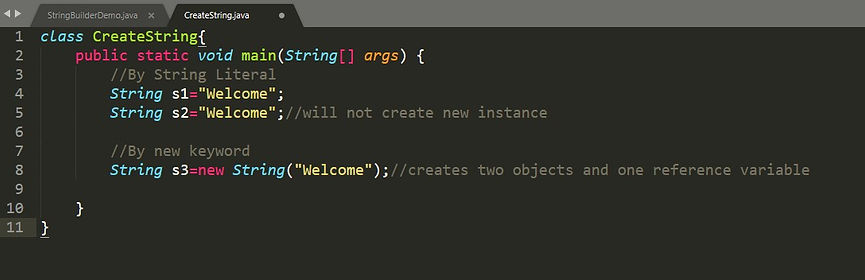
-
Java String class provides a lot of methods to perform operations on string
StringBuffer Class
-
It is same as String class except it is mutable i.e. it can be changed.
-
Java StringBuffer class is thread-safe i.e. multiple threads cannot access it simultaneously.
-
Some built-in methods of this class is synchronized.
StringBuilder Class
-
The Java StringBuilder class is same as StringBuffer.
-
It can build mutable string.
-
But it is non-synchronized.
-
Here is an example of StringBuilder methods in a program.
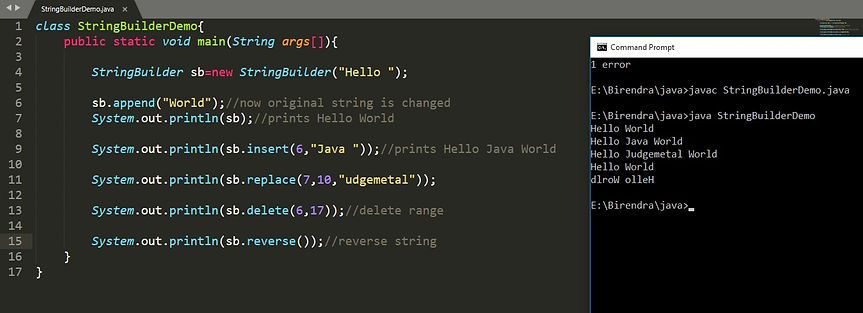
Program 1
-
Find the First non-repeated characher in a String.
-
Input: stoneprofits
-
Output: n
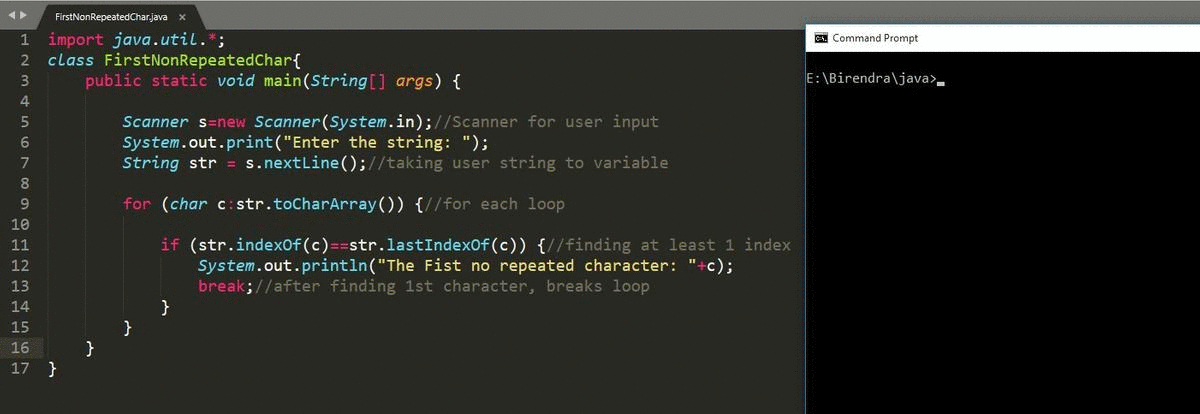
Program 2
-
Find Permutation of a Sting.
-
Input: ab
-
Output: ab, ab
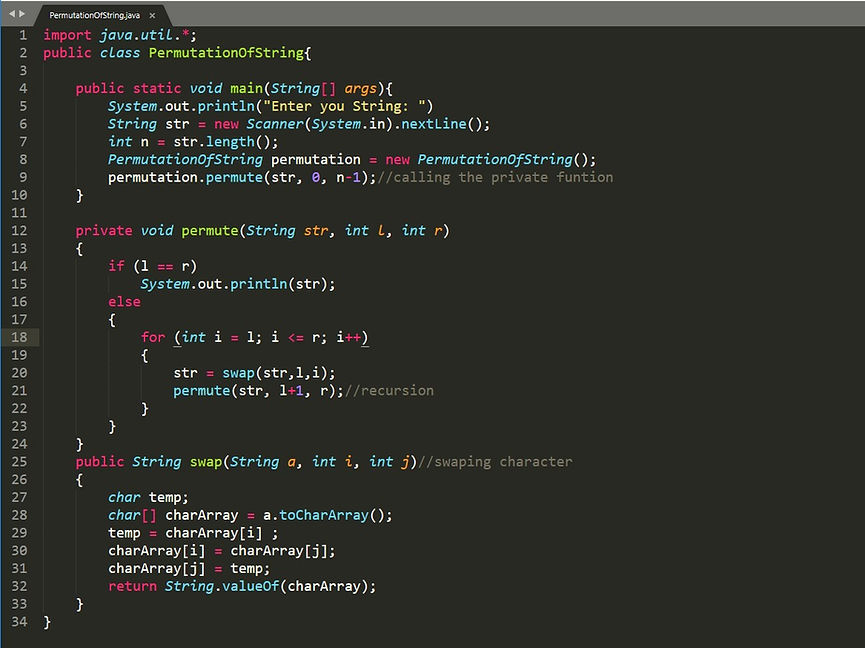
-
Output of the permutation programs is on below.
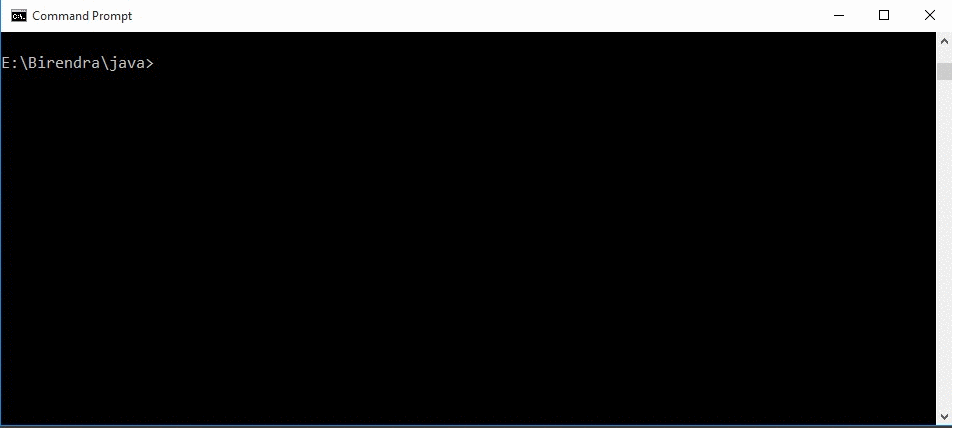
Program 3
-
Find Digit Identification if string have any number.
-
Input: stone123profits
-
Output: TRUE, it contains number.
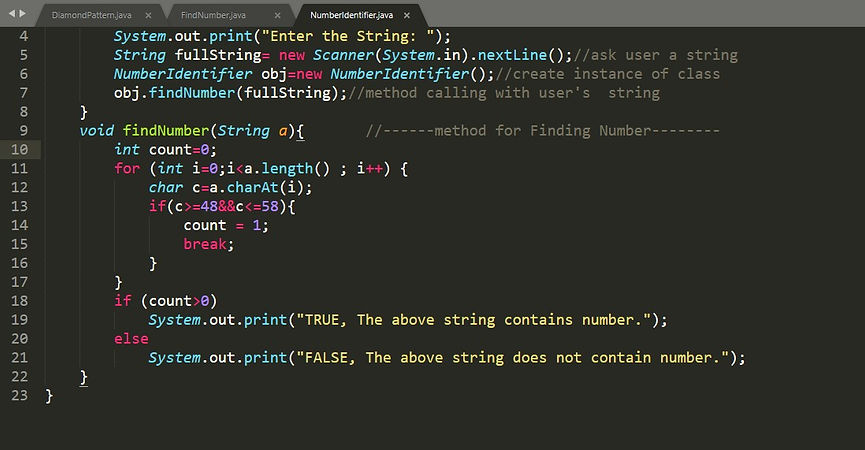
-
We checked here, with 2 string; one with number and another without number.
-
Here is the result shown in command prompt.
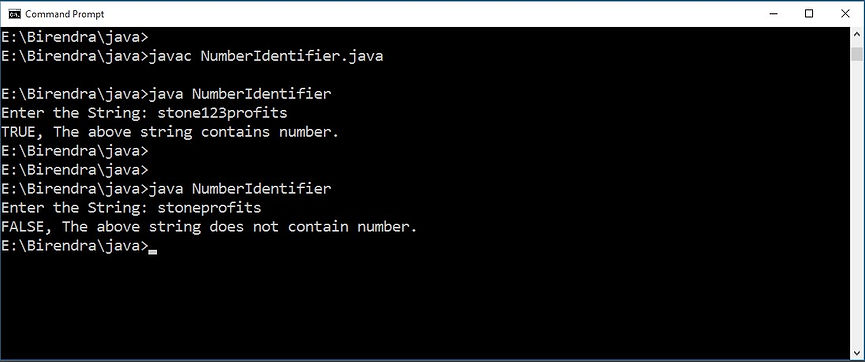
Program 4
-
Find & Replace the 1st highest repeated character with user given value.
-
Input: abcabab
-
Output: pbcpbpb
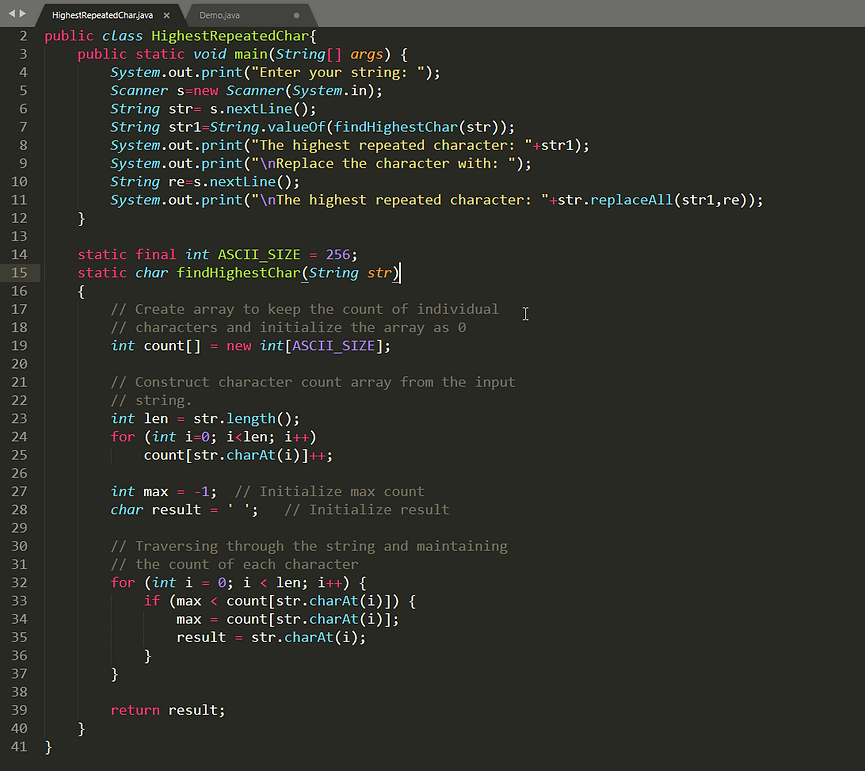
-
The output on Command Prompt looks like below.
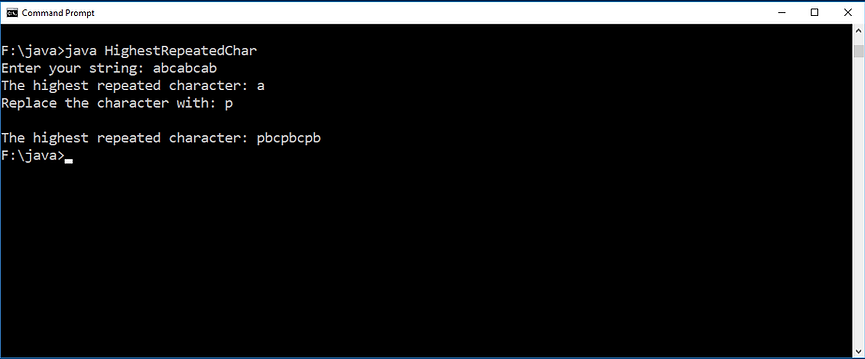
Program 5
-
WAP to print a Numbered Diamond.
-
Input: 5
-
Output: -

-
The Diamond output is here.
-
It asks a number as user input for make a diamond.
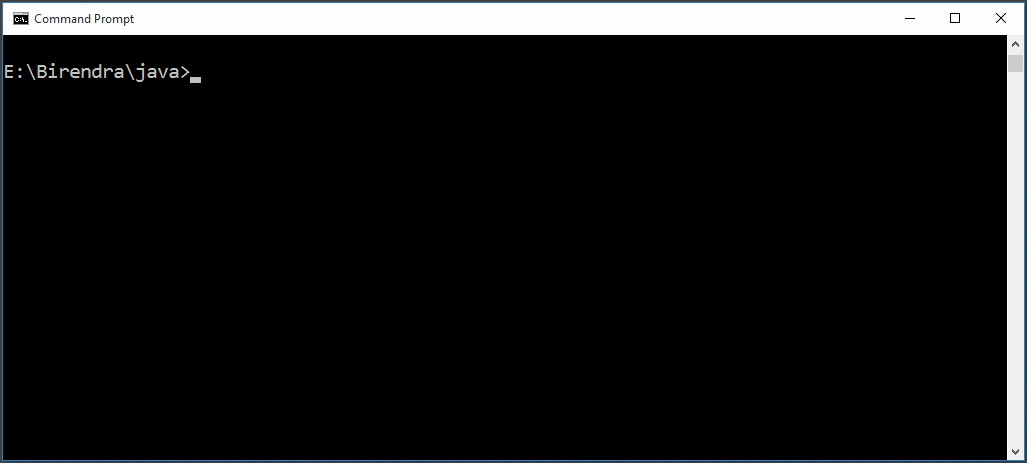
Program 6
-
WAP to sum the numbers in a String.
-
Input: stone123profits7
-
Output: 130
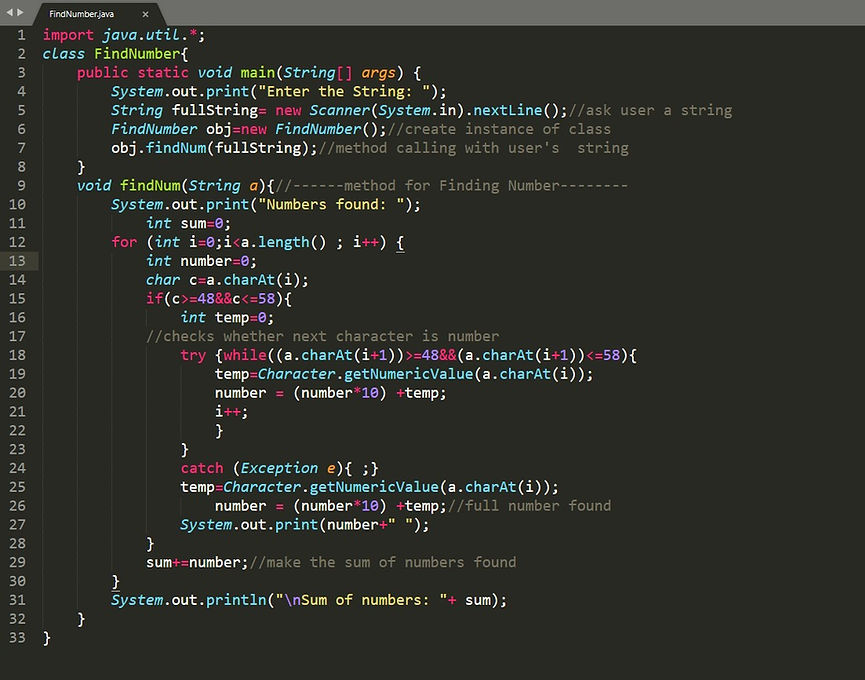
-
The output of FindNumber.java is executing in command prompt.
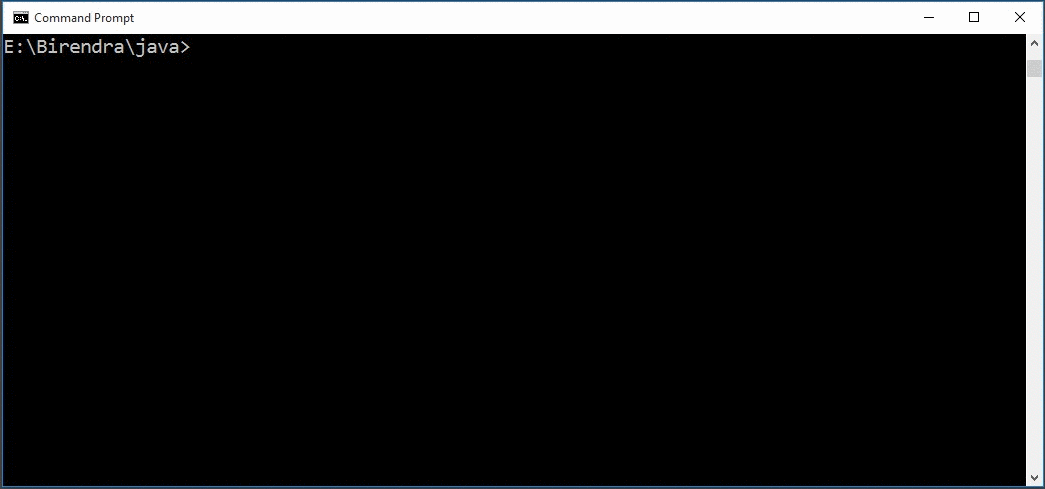
Java & Database
-
Java JDBC is a Java API to connect and execute a query with the database.
-
JDBC API uses JDBC drivers of respective RDBMS software to connect with the database.
-
There are 5 steps to connect any java application with the database using JDBC.
Step 1: Register the driver class
-
The forName( ) method of a Class class is used to register the driver class.
-
Used to dynamically load the driver class.
-
Syntax: Class.forName("com.mysql.jdbc.Driver")
Step 2: Creating a connection
-
The getConnection() method of DriverManager class is used to establish a connection with the database.
-
Syntax: Connection con=DriverManager.getConnection( "jdbc:mysql://localhost:3306/birenDB","system","password");
-
Wwhere jdbc is the API, mysql is the database, localhost is the server name.
-
BirenDB is the database name.
Step 3: Create a statement
-
The createStatement( ) method of is used to create a statement to execute queries with the database.
-
JDBC API provides 3 different interfaces to execute the different types of SQL queries.
-
Statement- Used to execute normal SQL queries.
-
PrepatedStatement- Used to execute dynamic or parameterized SQL queries.
-
CallableStatement- Used to execute the stored procedures.
-
Syntax: Statement stmt = con.createStatement();
Step 4: Executing Querry
-
executeQuery() method is used to execute a query.
-
It returns an object of ResultSet.
-
Syntax: ResultSet rs = stmt.executeQuery("select * from emp");
-
while(rs.next( )) { System.out.println(rs.getInt(1) + " " + rs.getString(2)); }
Step 5: Closing connection
-
For closing the Connection object & ResulSet object, we use close() method.
-
Syntax: con.close( );
Connector Driver
-
To connect any database, java need a respective driver for establishing a connection.
-
Now we have downloaded a mysql-connector.jar to make a connection between MySQL database.
-
There two ways to make to setup the connection:
-
Set as classpasth
-
or Paste the .jar file in C:\Program Files\Java\Jdk1.8.0_171\jre\lib\ext folder.
-
We kept the mysql-connector.jar file in ext folder.
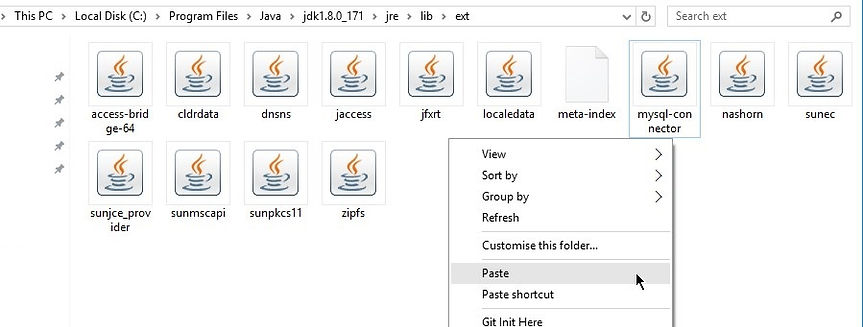
Program 7
-
WAP to Insert data into Database & retrive data from Databse.
-
Input: data
-
Output: data
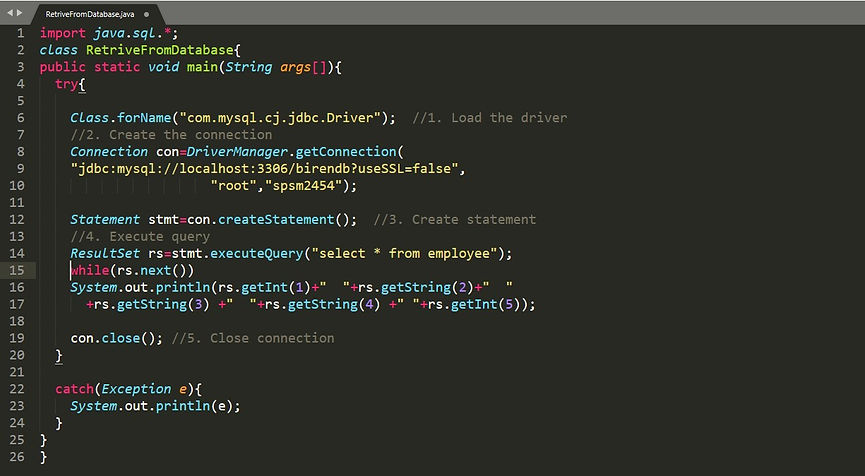
-
Here we wrote a Java program to retrieve the data from MySQL database.
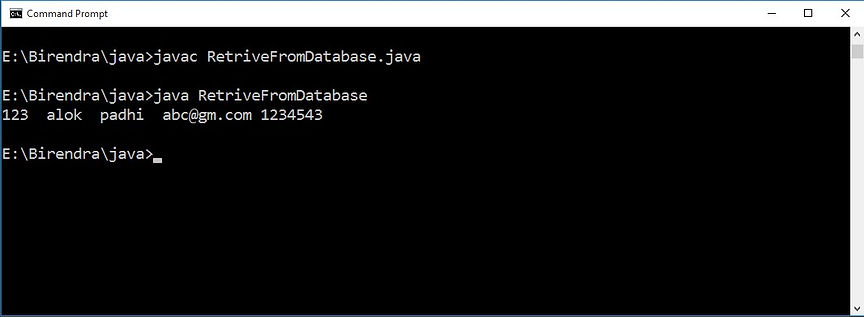
-
Here is an example of Inserting data into the Employee table in MySQL database.
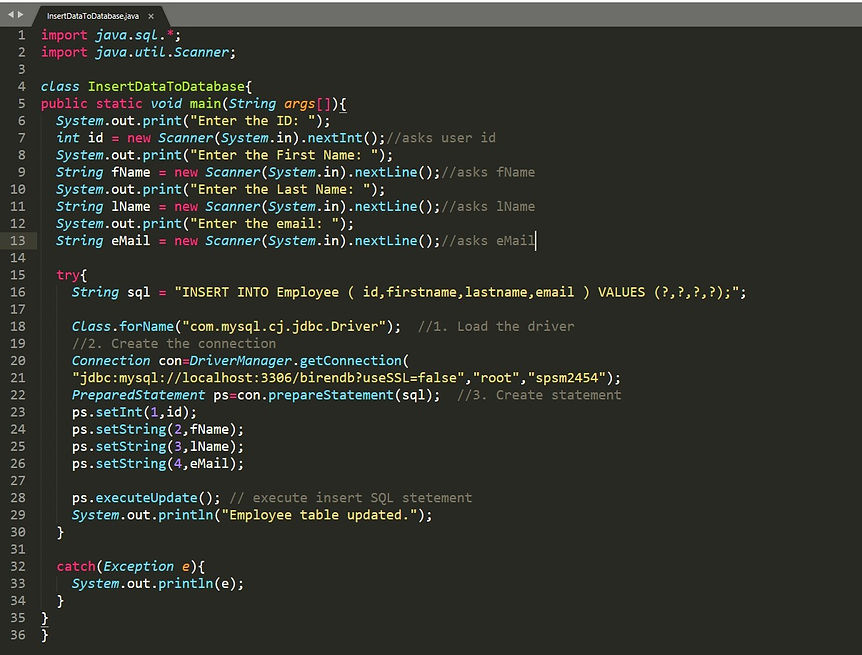
-
After compilation & execution of java program, we checked on MySQL database.
-
Here is the GIF output of before and after execution.
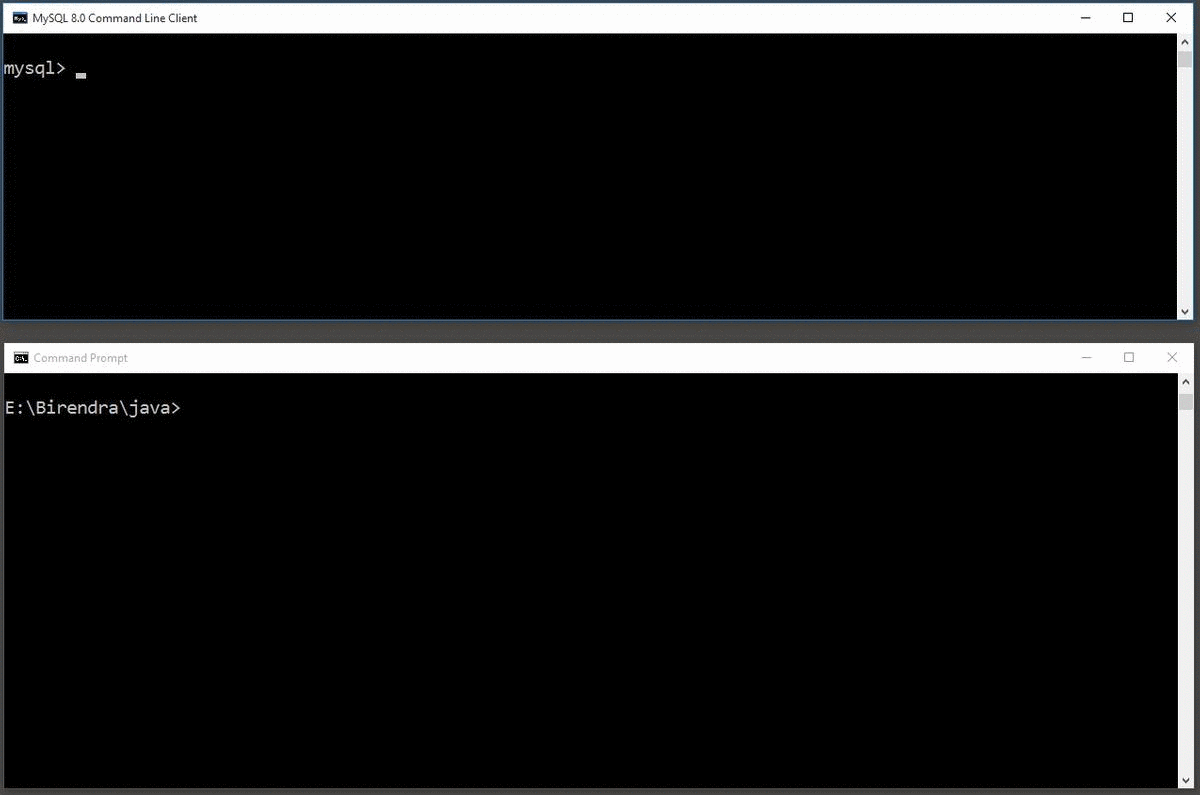
Write to a Excel File
-
For writing an excel file, we need to download some .jar file from apache official site.
-
Download the poi file from the website.
-
Extract & keep the jar files java jre/lib/ext folder.
-
These .jar files will help to write to an excel file.
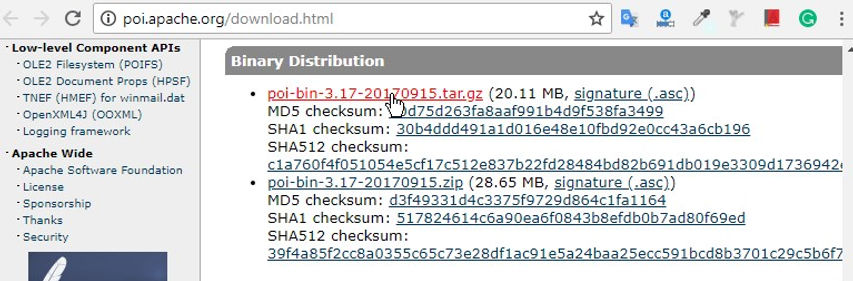
-
Here is the java program for writing to an excel file.
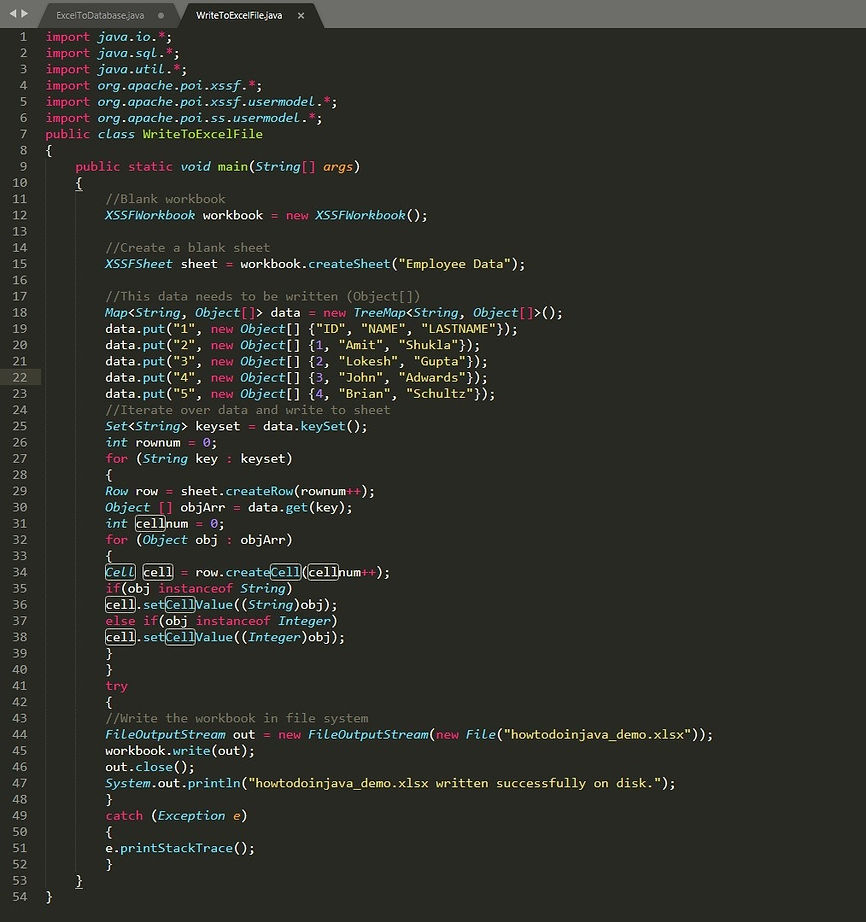
-
After executing the above program, we get a .xlsx file & opened on the google spreadsheet.
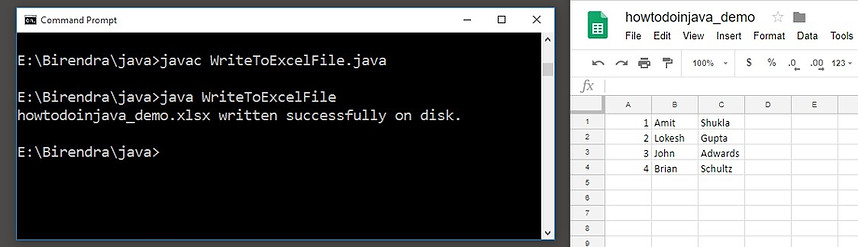
File Reading/Writing with Java
-
There are multiple ways of writing and reading a text file.
-
FileReader
-
BufferedReader
-
Scanner
-
BufferedReader provides buffering of data for fast reading.
-
But here we used Scanner for parsing and read line by line.
Exercise 4:
-
WAP to Read the 2nd & 4th line from a file.
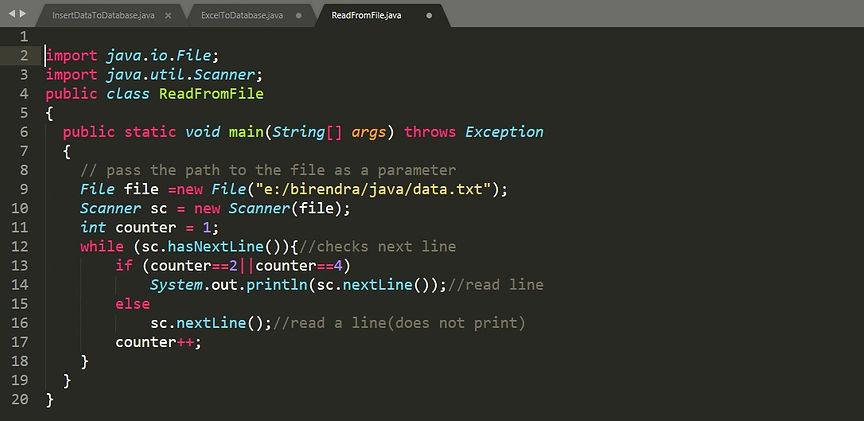
-
The data.txt file is located in e:/birendra/java path.
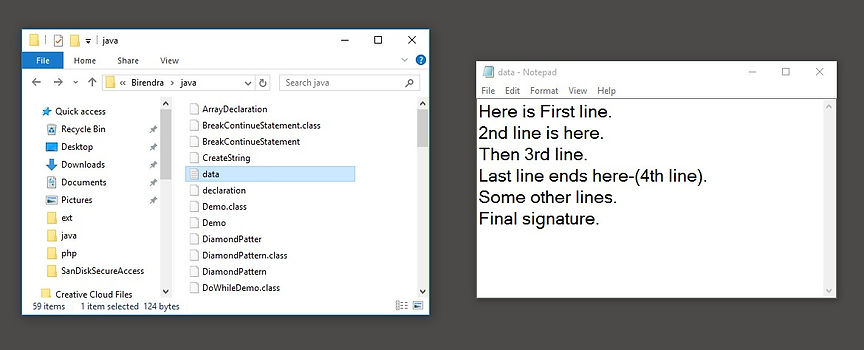
-
After executing the ReadFromFile.class file, here it reads 2nd & 4th line form a text file.
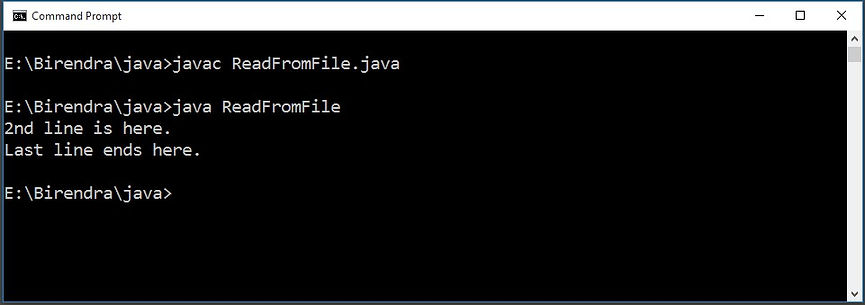
Write to File
-
To write to a file we used FileOutputStream, OutputStreamWriter, File & ButterWriter class.
-
These all are from java.io package.
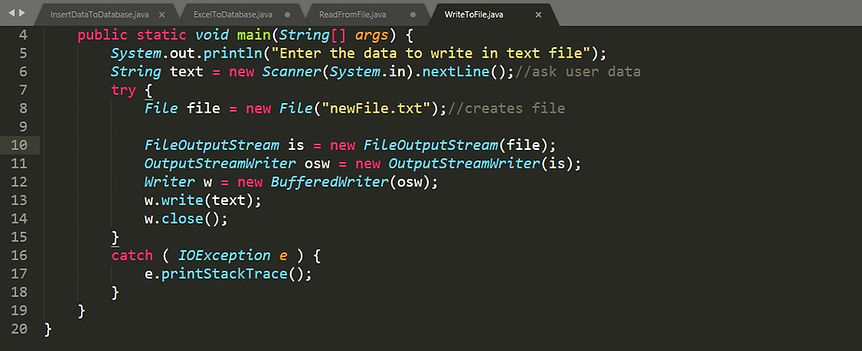
-
Here is the example of writing to a text file.
-
It creates a new file or overrides the file with the user ented string.
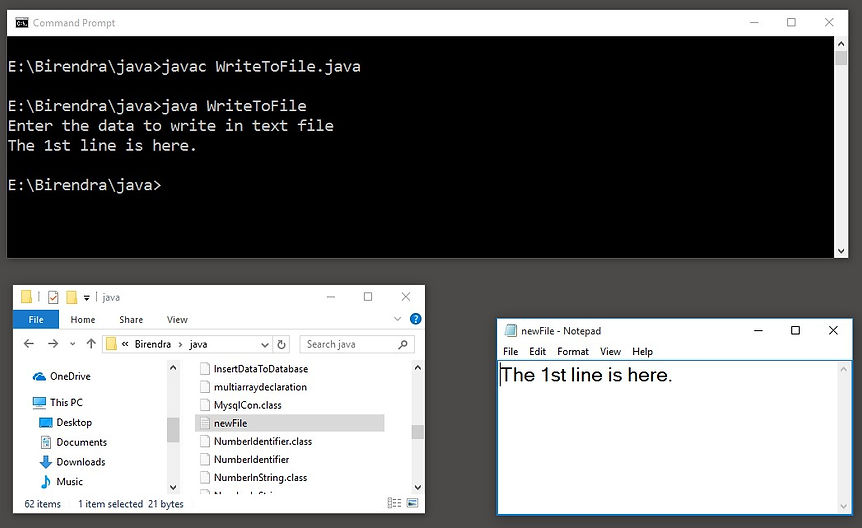
Graphics In Java
-
JAVA provides a set of libraries to create Graphical User Interface.
-
To achieve this functions, we need 3 packages:
-
AWT: Platform specific.
-
Swing: Only for a desktop application.
-
JavaFX: Desktop, Website, and Handheld device friendly.
-
Here we wrote a program for drawing some geometric shapes; a line, a rectangle, a circle, a square.
-
The below java program has imported java.awt & javax.swing packages for making a Graphics &Frame respectively.
Exercise 6:
-
WAP to draw a line, square, rectangle & a circle.
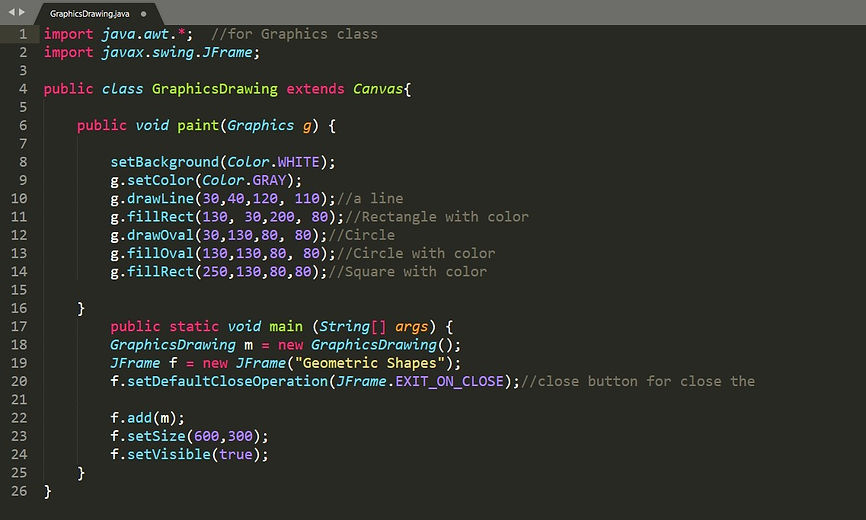
-
After compilation & execution of the program, a window will pop up, is known as Fame.
-
All the shapes will be shown here.
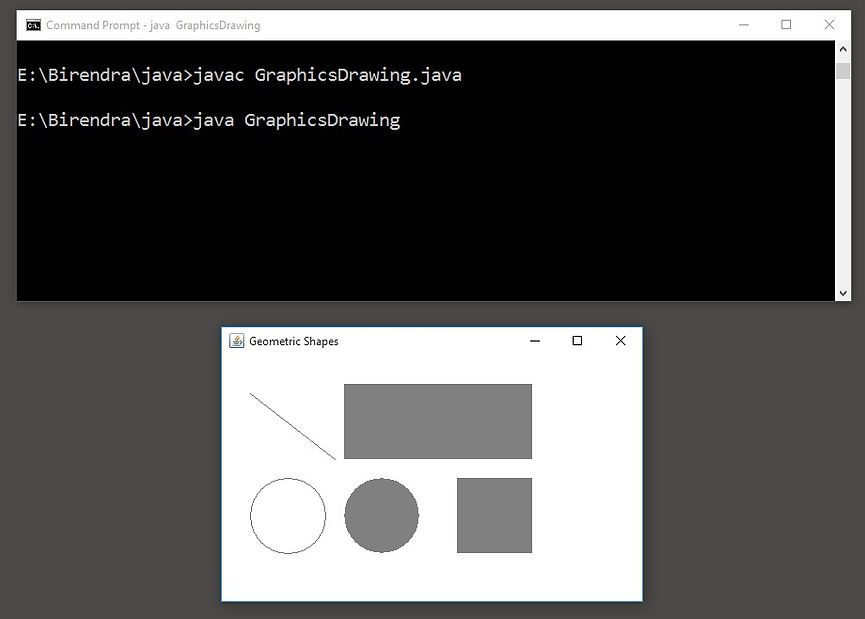
bottom of page