top of page
PHP
What is it?
-
PHP is a server scripting language.
-
It is used for making dynamic and interactive Web pages.
-
PHP stands for Hypertext Pre-processor.
-
Both PHP and JavaScript can be embedded into HTML pages.
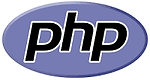
Wha is a PHP file?
-
PHP file extension is ".php"
-
A PHP file can contain text, HTML, CSS, JavaScript, and PHP code.
-
It executed on the server.
-
The result is returned to the browser as plain HTML.
-
A script is a set of programming instructions that are interpreted at runtime.
-
The purpose of the scripts is usually to enhance the performance or perform routine tasks for an application.
-
PHP is a case-sensitive language, “VAR” is not the same as “var”.
Why PHP?
-
Runs PHP file extension is ".php"
-
A PHP file can contain text, HTML, CSS, JavaScript, and PHP code.
-
It executed on the server.
-
The result is returned to the browser as plain HTML.
What is XAMPP?
-
XAMPP is an open source cross-platform web server,
-
It contains MySQL database engine, and PHP and Perl package.
-
It is compiled and maintained by apache.

X A M P P
cross-platform operating systems
Apache
MySQL
PHP
Perl
XAMPP deals with all the complexity in setting up and integrating with Apache, PHP, MySQL, and Perl.
Why use XAMPP?
-
In order to use PHP, you will need to install PHP, Apache and maybe even MySQL.
-
It’s not easy to install Apache and configure it.
-
XAMPP deals with all the complexity in setting up and integrating with PHP and Perl.
-
Unlike JAVA, PHP needs a web server to work.
-
XAMPP provides an easy to use the control panel to manage:
How to Download and Install XAMPP?
-
Here are the steps to install XAMPP for Windows.
-
Download the XAMPP installer here. https://www.apachefriends.org/download.html
-
If you are getting a warning message "Important! Because of an activated User Account Control (UAC) on your system".
-
Make sure you deactivate the UAC feature.
-
To do this, Select Control Panel > User Account > Change Access Control setting > Set the meter as lowest > OK.
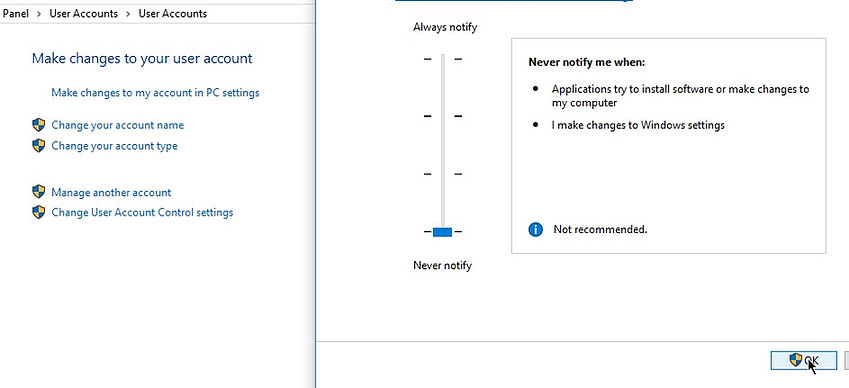
-
In the next screen, Change the installation path if required. Click Next.
-
In the next screen select Apache and MySQL.
-
Before we test our XAMPP installation, let’s first look at the basic directories that we will be working with.
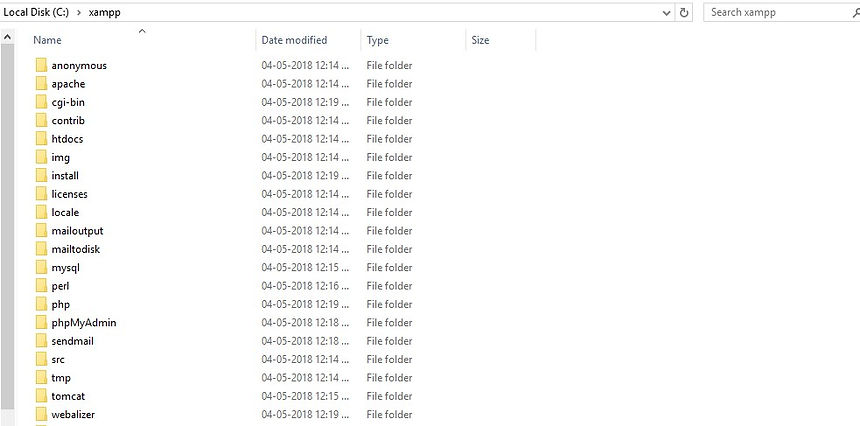
-
mysql: This directory contains all the information related to MySQL database engine, by default port no. 3306.​​
-
htdocs: This is the web root directory. All of our PHP codes will be placed in this directory
-
php: This directory contains PHP installation files. This directory is used to configure how PHP behaves on your server.
-
Start the XAMPP Control Panel, which looks like a below pic.
-
Apache, MySQL server and Tomcat service have been installed.
-
Green highlight represents, Apache has started.
-
Red highlight represents, here MySQL and Tomcat has not started, they got some issue.

-
Look at the basic configurations required before we start using our XAMPP installation for developing PHP powered websites.
-
Type the URL http://localhost/xampp/ in your favorite browser.
Hello PHP Program
<!DOCTYPE html>
<html>
<body>
<?php
echo "My first PHP script!";
?>
</body>
</html>
-
Here I installed Adobe Dreamweaver IDE, where I will keep the php and HTML coding.
-
The below screenshot of Dreamweaver IDE, and the Hello PHP program.​

Output on Browser
PHP code in editor
Setup PHP on IIS (Internet Information Services)
-
If you have an issue with XAMPP server.
-
You can setup PHP on IIS, a Windows application.
-
Download PHP package form here, https://windows.php.net/download#php-7.2 .
-
Extract the zip file > Rename the folder to PHP > Keep that folder on C.
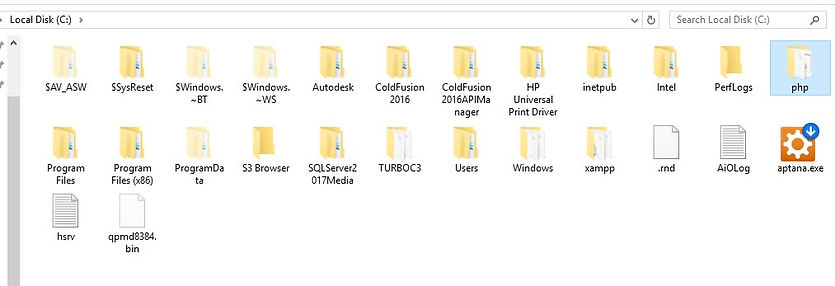
Keep PHP folder on C drive
-
Change the file name; php.ini.production to php.ini > Save.​
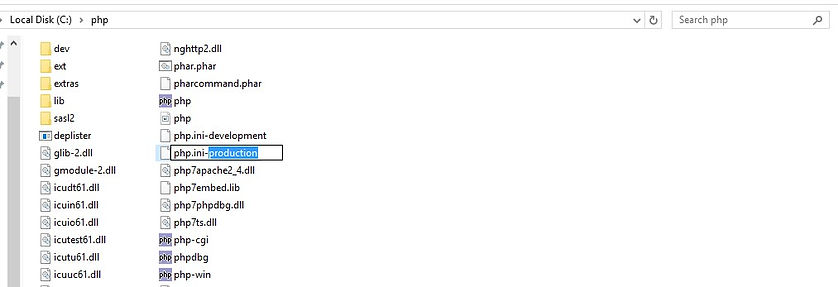
Rename the file as php.ini
-
Open that file (php.ini) in notepad.
-
Find extension_dir = , set the value as "c:\php\ext".
-
Remove ; or semicolon before 3 parameters; extension_dir, cgi.force_redirect, & fastcgi.impersonate.
-
Save that file.
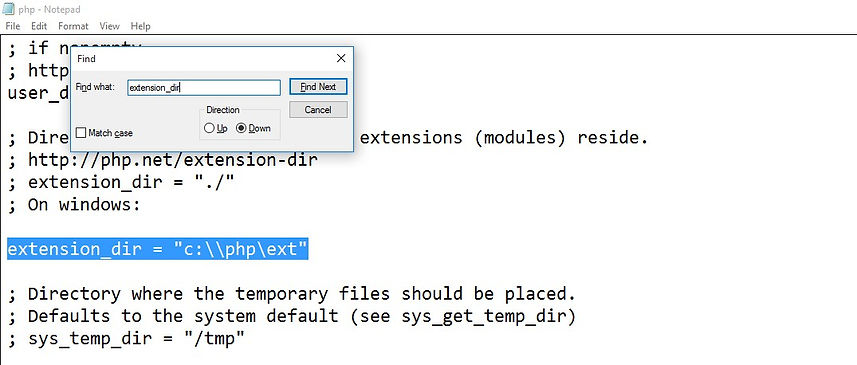
-
Open IIS (Internet Information Services).
-
Click on Default Document on IIS > Open feature > Add > index.php > OK.
-
Click on Directory Browsing > Open feature > Enable.
-
Click on FastCGI Setting > Open feature > Add Application > set full path "c:\php\php-cgi.exe" > Open > OK.
-
Click on Handler Mapping > Open feature > Add Module Mapping > set the followings.
-
Request path: *php
-
Module: FastCGIModule
-
Executable: php.cgi.exe
-
Name: PHP
-
Save
-
Restart the server.
-
Create a new site > set a physical path where the PHP pages we will make.
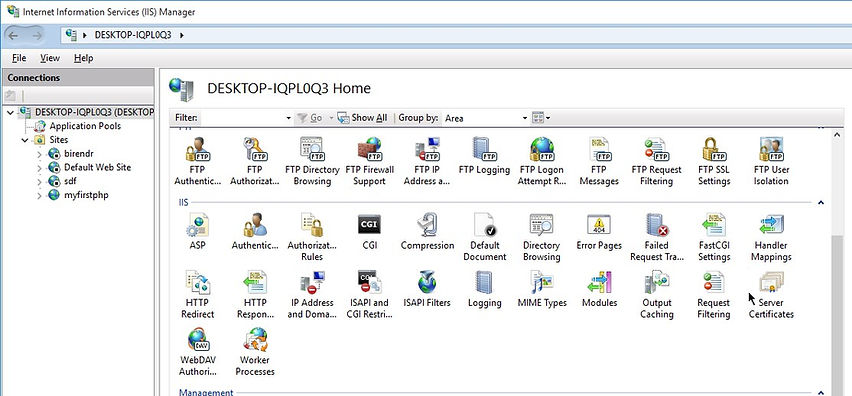
Add some features
-
Type a hello PHP code > save as .php > in D:\\PHP folder.
-
Open browser > type localhost/php
-
Here are our hello php page looks in a browser.
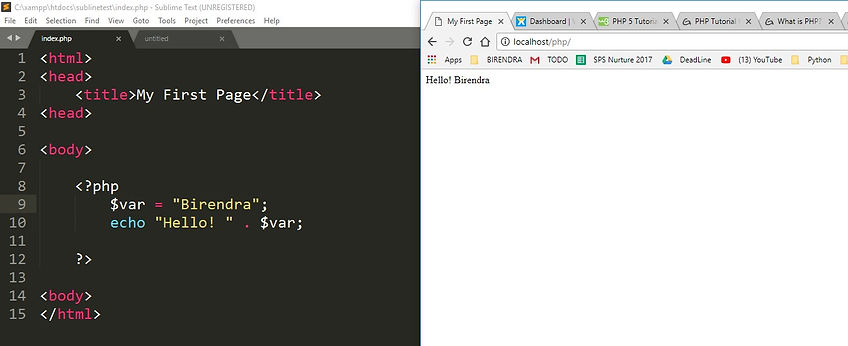
PHP Data Types
-
A datatype is the classification of data into a category according to its attributes.
-
A variable is a name given to a memory location that stores data at runtime.
-
String
-
Integer
-
Float
-
Boolean
-
Array
-
Object
-
PHP determines the data type depending on the attributes of the supplied data.
Variable Initialization Rules
-
A Php global variable is accessible to all the scripts in an application.
-
A local variable is only accessible to the script that it was defined in.
-
All variable names must start with the dollar sign ( $ ).
-
eg. $var, $var1, $var_one, etc.
-
Here are some rule followed when creating variables.
-
All variables names must start with a letter followed by other characters.
-
Variable must not contain space.
-
We can not use dollar ( & ) or minus sign( - ) to separate variable names.
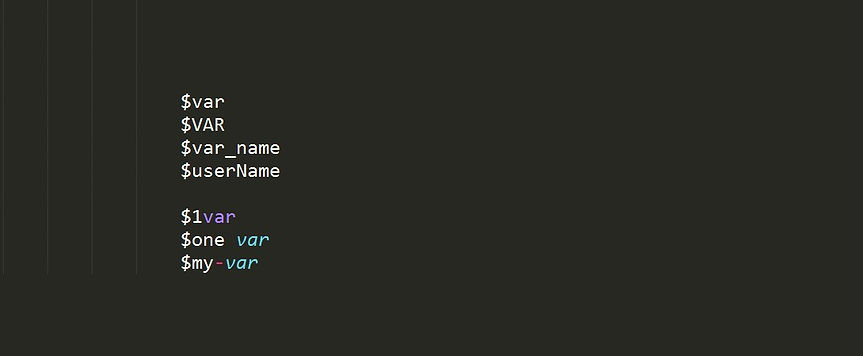
Correct way of defining variable
Incorrect way of defining variable


-
Integer numbers
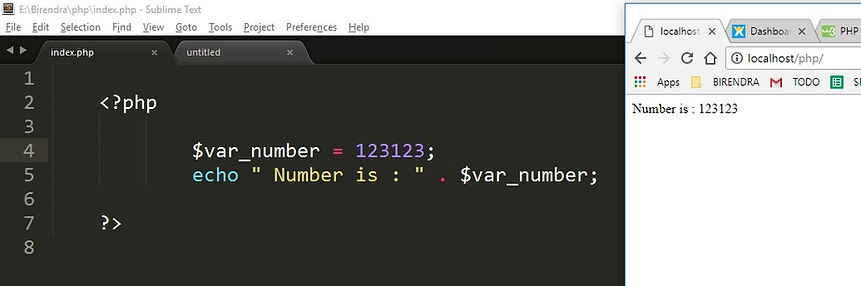
-
Floating point numbers
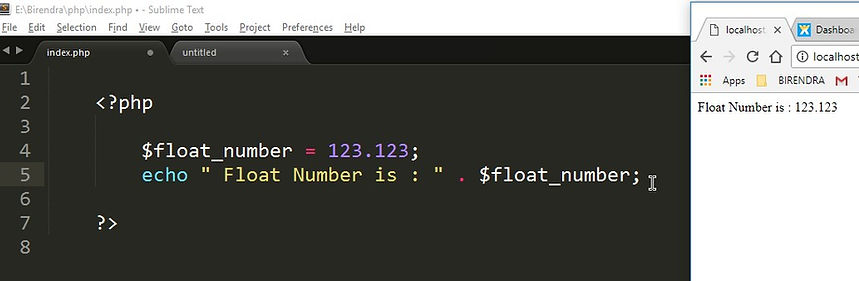
-
Character strings
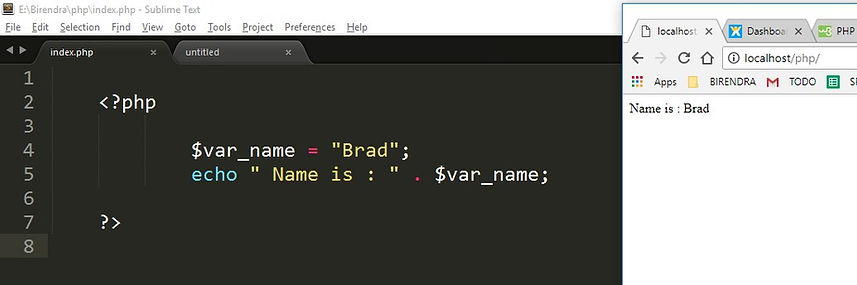
-
Character strings
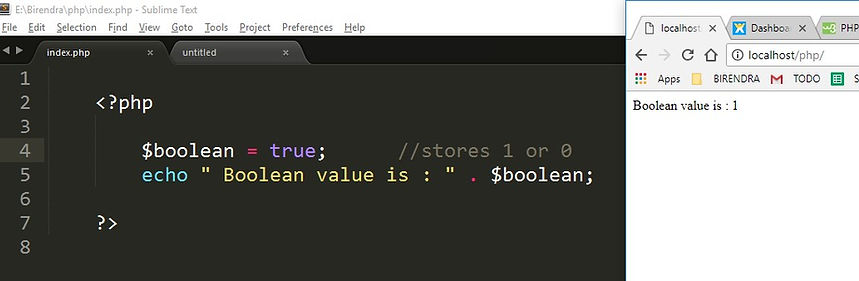
-
Array of String
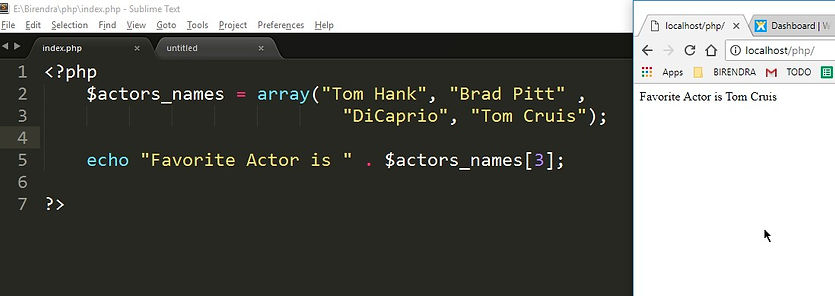
Variable Type Casting
-
Typecasting is converting a variable or value into the desired data type.
-
This is very useful when performing arithmetic computations that require variables to be of the same data type.
-
Typecasting in PHP is done by the interpreter.
-
The var_dump function is used to determine the data type
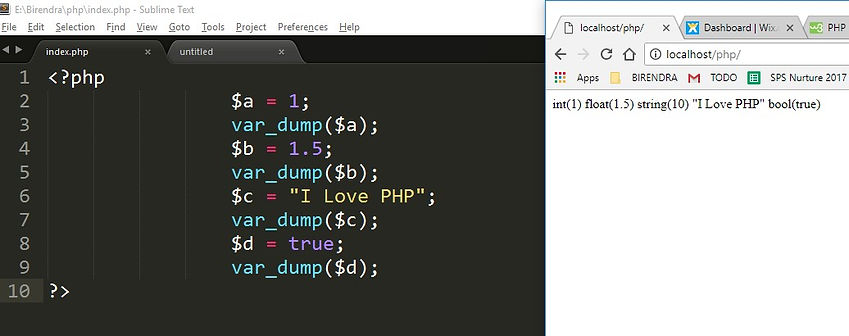
PHP Constant
-
A constant is a variable whose value can not be changed at runtime.
-
We can a value to a variable, that never chages.
-
Syntax: define ( 'PI' , 3.14 ) ;
-
It creates a constant with a value of 3.14.
-
We can not assign a different value.
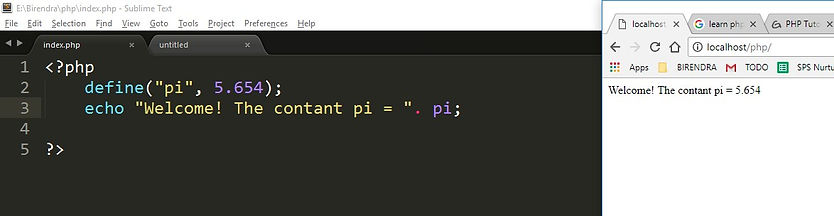
PHP Arithmatic Operator
-
Arithmetic operators are used to performing arithmetic operations on numeric data.
-
The concatenate operator works on strings values too.
-
PHP supports the following operators.
PHP Assigning Operator
-
Assignment operators are used to assigning values to variables.
-
They can also be used together with arithmetic operators.
PHP Comparison Operator
-
Comparison operators are used to compare values and data types.
PHP Logical Operator
-
Logical operators are used to compare conditions or values.
-
When working with logical operators, any number greater than or less than zero (0) evaluates to true.
-
Zero (0) evaluates to false.
Comments
-
Commenting the source code helps remember what the code does.
-
Can be easily understood by other developers by simply reading the comments.
-
Single line comments start with double forward slashes // and they end in the same line.
-
Multiple line comments start with a forward slash followed by the asterisk /* and end with the asterisk followed by the forward slash */.
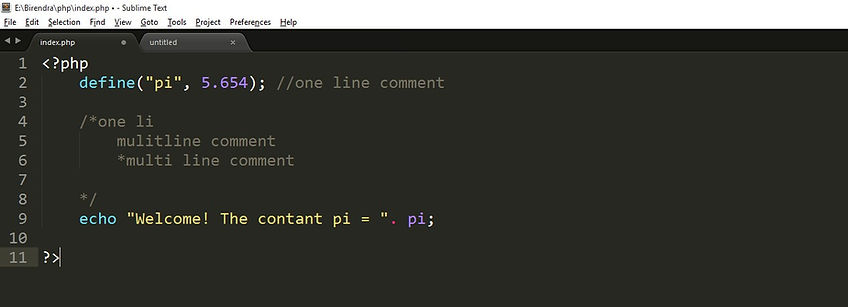
Include/Include_once
-
The “include” php statement is used to include other files into a PHP file.
-
It has two variations, include and include_once.
-
Include_once is ignored by the PHP interpreter if the file to be included.
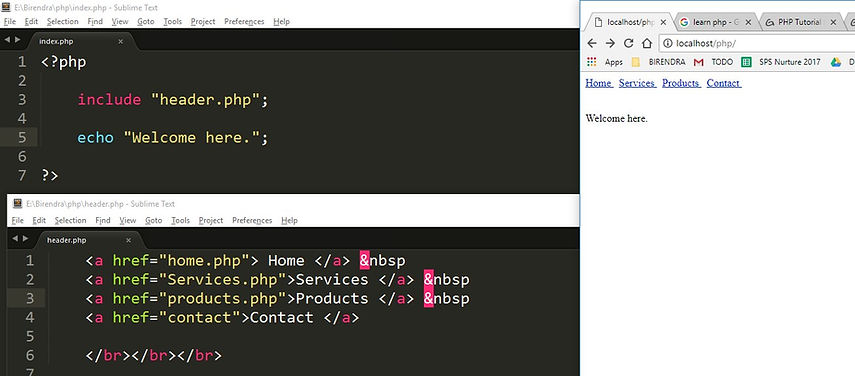
Require/Require_once
-
The require/require_once statement is used to include file.
-
Require_once is ignored if the required file has already been added by any of the four include statements.
PHP Array
-
An array is a special variable, which can hold more than one value at a time.
-
We can access the values by referring to an index number.
-
In PHP, the array() function is used to create an array.
-
Here, the array is of 3 types.
PHP Array
Indexed Array
Arrays with a numeric index.
Associative Array
Arrays with named keys.
Multi-dimensional Array
Arrays containing one or more arrays.
Indexed Array
-
The index can be assigned automatically (index always starts at 0).
-
Or the index can be assigned manually.
-
count() function is used to know the length of the array.
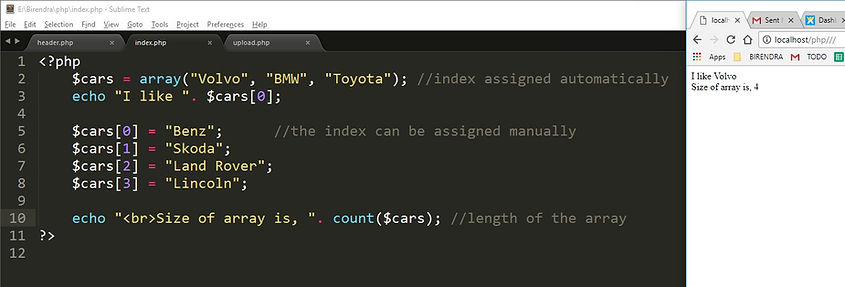
Associated Array
-
Information can be passed to functions through arguments. An argument is just like a variable.
-
The following example has a function with two arguments ($fname and $year):
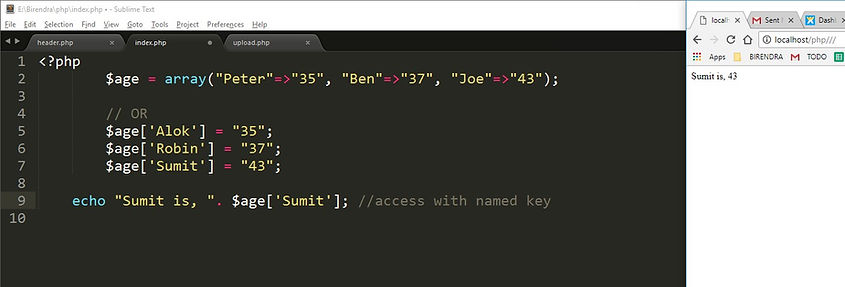
Multi-Dimensional Array
-
A multidimensional array is an array containing one or more arrays.
-
The dimension of an array indicates the number of indices you need to select an element.
-
PHP understands multidimensional arrays that are two, three, four, five, or more levels deep.
-
For a two-dimensional array, you need two indices to select an element.
-
For a three-dimensional array, you need three indices to select an element.
-
The advantage of multidimensional arrays is that they allow us to group related data together.
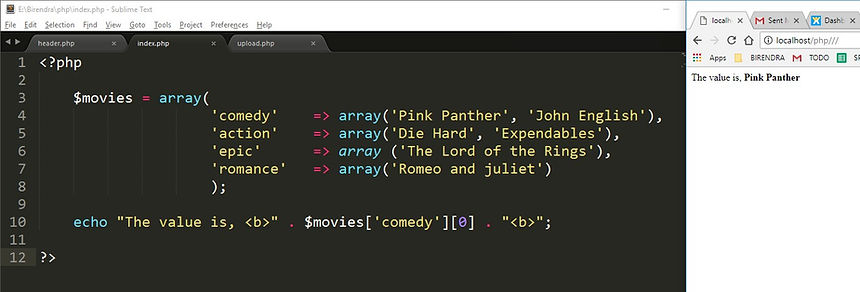
-
There are lots of built-in functions in PHP.
-
This allows to access and manipulate the arrays.
-
Arrays easily help group related information such as server login details together.
PHP Functions
-
We can create a function.
-
PHP allows us to reuse the code over and over again.​
-
A function will be executed by a call to the function.
-
Function names are NOT case-sensitive.
-
It has more than 1000 built-in functions.
Syntax:
function addNumbers ( $num1 , $num2 ){
​
return num1 + $num2 ;
}
​
echo " 3 _+ 4 = " . addNumber ( 3 , 4 );
Functions Arguments
-
Information can be passed to functions through arguments. An argument is just like a variable.
-
The following example has a function with two arguments ($fname and $year):
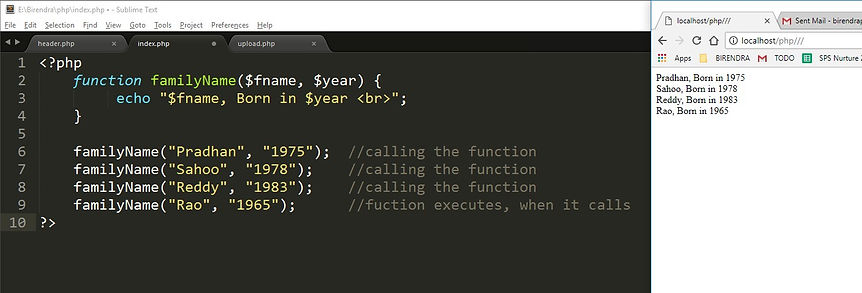
Function with Default Argument Value
-
If we call the function without arguments it takes the default value as an argument
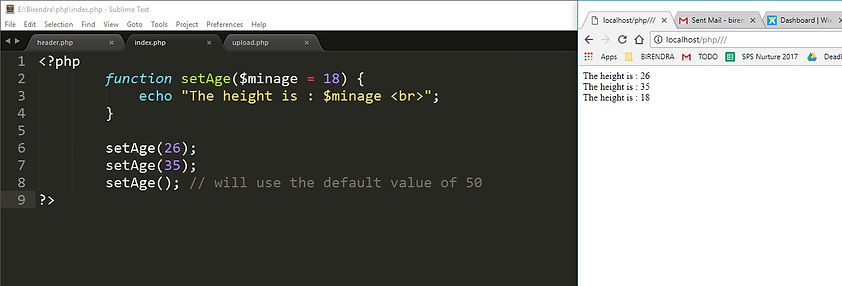
Function with Returning values
-
To let a function return a value, use the return statement.
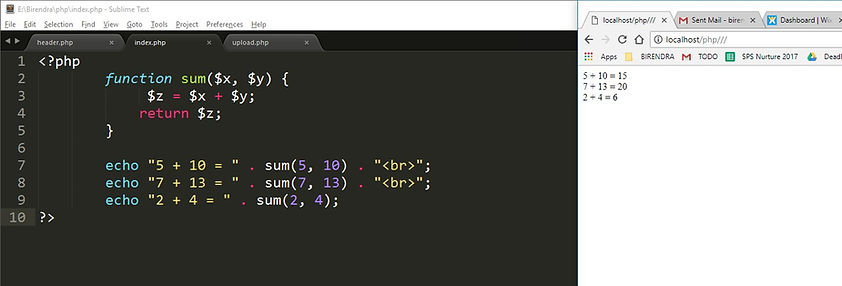
Mathematical Functions
-
The math functions can handle values within the range of integer and float types.
-
The PHP math functions are part of the PHP core.
-
There are a number of functions available related to numerical and mathematical.
-
We cover some important functions.
PHP String
-
A string is a collection of characters, can contain alphanumeric characters.
-
It is one of the data types supported by PHP.
-
​Strings are created when;
-
We declare variable and assign string characters to it.
-
Use them with echo statement.
Create Stings
-
The simplest way to create a string is to use single quotes.
-
The double quotes are used to create relatively complex strings compared to single quotes.
-
Variable names can be used inside double quotes and their values will be displayed.
-
To show any character like ' , " , \ or any character, use the backslash " \ " before character.
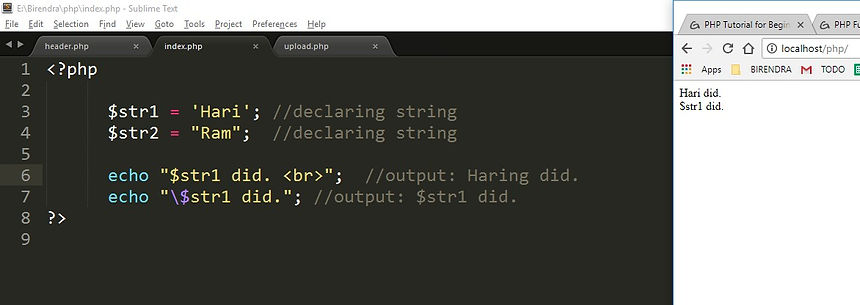
PHP Heredoc
-
Heredoc method is used to create string values.
-
You can also use double quotes inside without escaping them.
-
<<<EOT (end of the text) is the string delimiter.
PHP Nowdoc
-
Nowdoc is an identifier with a single quote string.
-
No parsing is done inside a Nowdoc.
-
Nowdoc is supported only by PHP 5.3.
-
Can not pass the variable.
-
strstr ( $randString, 'Ravi' ); This function searches for the occurrence of a string inside another string. It returns the rest of the string (from the matching point), or FALSE, if the string to search for is not found.
PROGRAM 2
-
Write a program to count the no. of occurrence of the substring in a bigstring.
-
Here the full string is $i, and the substring is $subString.
-
The output is 4.
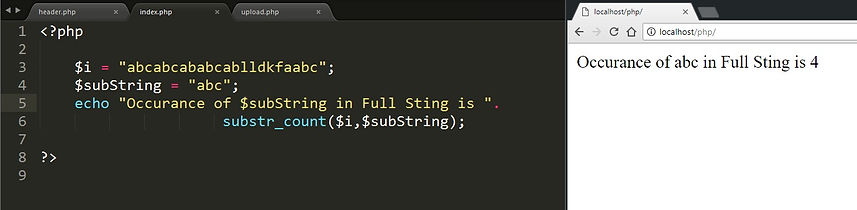
Conditional Execution
-
Conditional statements are used to perform different actions based on different conditions.
-
In PHP we have the following conditional statements:
-
if statement - executes some code if one condition is true.
-
if...else statement - executes some code if a condition is true and another code if that condition is false.
-
if...elseif....else statement - executes different codes for more than two conditions.
-
switch statement - selects one of many blocks of code to be executed.
IF statement
-
You have a block of code that should be executed only if a certain condition is true
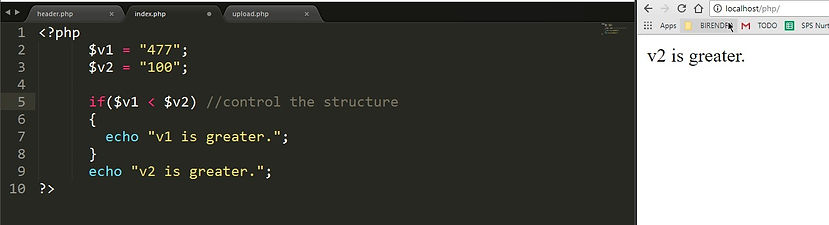
IF...ELSE statement
-
ou have two options, and you have to select one.
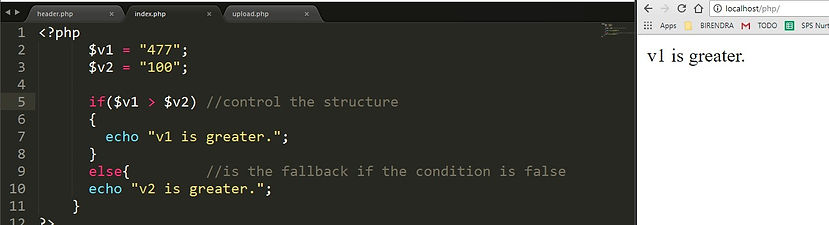
IF...ELSEIF...ELSE statement
-
when you have to select more than two options and you have to select one or more
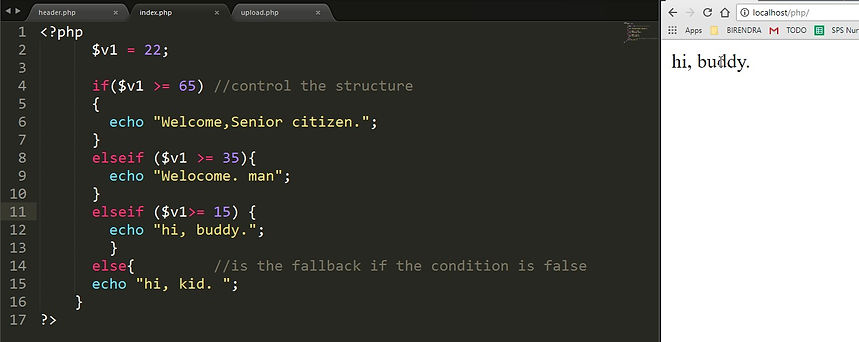
SWITCH statement
-
Switch… case is similar to the if then… else control structure.
-
It only executes a single block of code depending on the value of the condition.
-
If no condition has been met then the default block of code is executed.

Loop Execution
-
Conditional statements are used to perform different actions based on different conditions.
-
In PHP we have the following conditional statements:
Loop Types
FOR
for..each
WHILE
while
do...while
for
FOR loop
-
For loops execute the block of code a specified number of times.
-
In for loop syntax, there are 3 factors are there:
-
initialize: usually an integer.
-
condition: if the condition is true, the loop continues.
-
increment: increment the value of an integer.
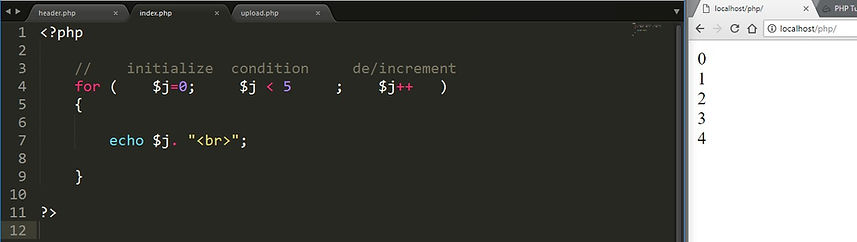
FOR...EACH loop
-
Foreach loop is used to iterate the array values.
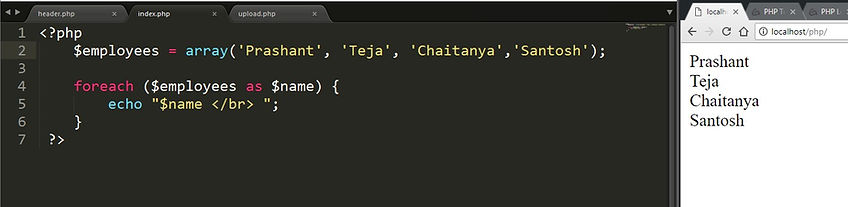
-
Another example for an associative array.
-
Here we declare key and value as a variable & use them.
-
An associative array uses alphanumeric words for access keys and value.
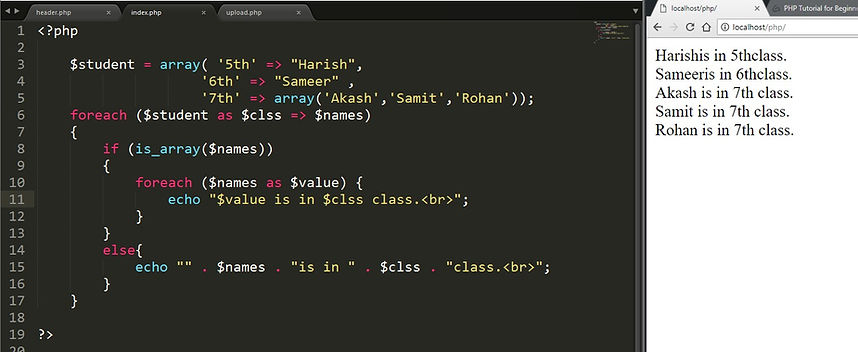
WHILE loop
-
It is used to execute a block of code a repeatedly until the set condition gets satisfied.
-
In While loop, it checks the condition first. If it evaluates to true, the block of code is executed as long as the condition is true.
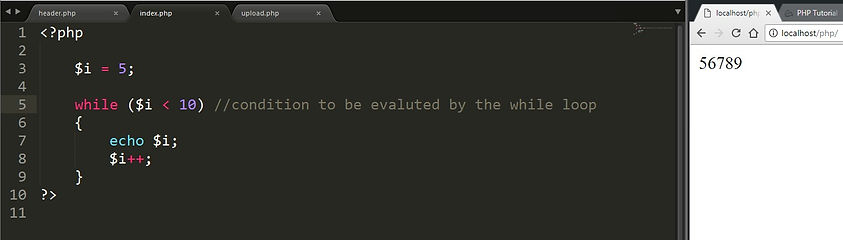
DO...WHILE loop
-
Here it executes the block of code at least once before evaluating the condition
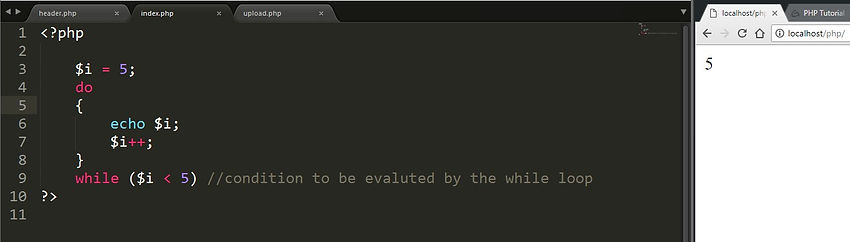
Reading Files into a String or Array
-
Here we will see how to read/write a file.
-
There are some funtion in PHP, which allows us to work with an entire file as a single variable.
-
There are several ways for reading a file.
Method 1
-
Include or Requre puts the content of the specified file directly into the current file as if you had typed it yourself.
include 'myfile.txt';
Method 2
-
Open a connection to a file, using fopen( ).
-
Work with that file line by line before you close the resource.
-
Funtions for reading each line are fgetc, fgets, fgetss, fread, and fwrite.
if ( $fh = fopen ( 'mytext.txt' , 'r' ) ) {
while ( !feof ( $fh) ) {
$line = fgets ( $fh ) ;
echo $line ;
}
fclose ( $fh ) ;
}
Method 3
-
Read the entire file into a string using file_get_contents.
$file = file_get_contents ( 'myfile.txt' ) ;
echo $file ;
Method 4
-
Read the entire file into an array of lines using file.
$file_lines = file ( 'mytext.txt' ) ;
foreach ( $file_lines as $line ) {
echo $line ;
}
Write a File
-
The fopen() function is also used to create a file.
-
Here is an example of PHP (writeFile.php) for writing data to a text file.
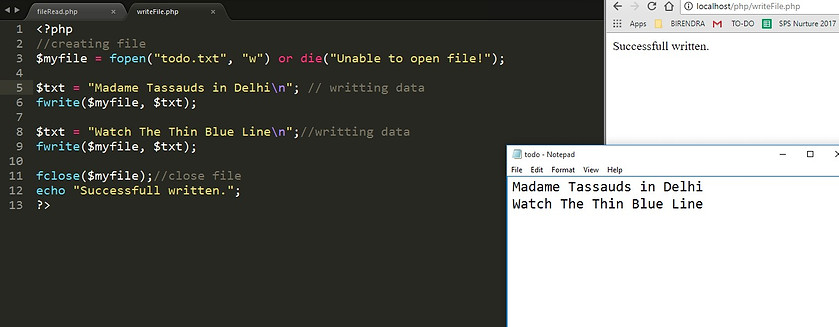
Cookies
-
A cookie is a small file, that the web server stores on the client computer.
-
Once the cookie has been set, all page requests that follow return the cookie name and value.
-
Maximum size: 4kb.
-
A cookie created by a user can only be visible to them.
-
Other users cannot see its value.
-
The web browser has option for disabling cookies & third party cookies.
Why Cookies
-
Tracks the states of the application.
-
Personalize the user experience.
-
Tracking the pages visited by a user.
Creating Cookies
-
In PHP, setcookie() is a function used to create a cookie.
-
$_COOKIE is array variable, the server uses for retrieving its value.
-
Syntax: setcookie(cookie_name, cookie_value, [expiry_time], [cookie_path], [domain], [secure], [httponly]);
Retriving Cookies Value
-
$_COOKIE is used to retrieve data.
-
To show the content of the cookie array variable, syntax: print($_COOKIE);
-
The default size of the cookie is 1 GB, can be changed in the php.ini file.
Delete Cookies
-
To destroy a cookie before its expire time, we can set a expire time with negative (-ve) sign.
-
Syntax: setcookie("user_name", "Tom", time() -3600, ' / ');
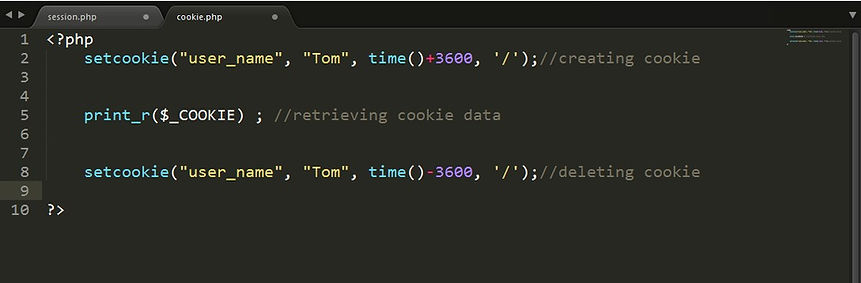
Session
-
A Session is a global variable stored on the server.
-
Each session is assigned a unique id which is used to retrieve a value.
-
Session id is stored in a $_COOKIE global array variable & $_SESSION.
-
We can store important user data in the session.
-
Here is the syntax of creating & destroying the session.
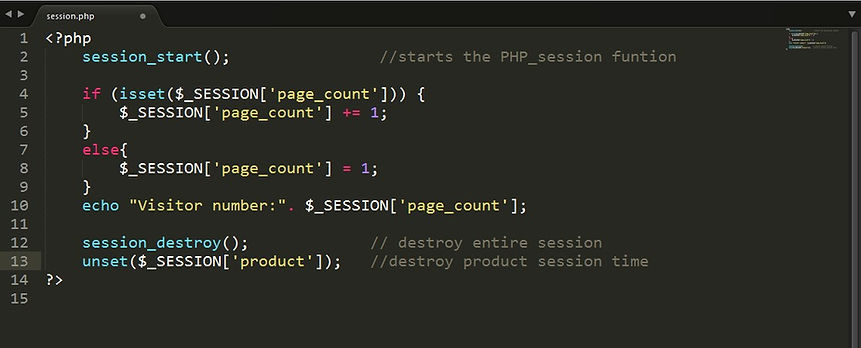
PHP & MySQL Database
-
With PHP, we can connect to a database and manipulate the database.
-
MySQL is the most popular database used with PHP.
-
The data in a MySQL database are stored in tables.
-
A table is a collection of related data, it consists of rows and columns.
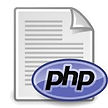
insert & retrieve data
.php file
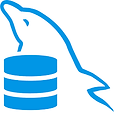
MySQL Database
-
PHP 5 and later works with a MySQL database using:
-
MySQLi extension( i mens improved)
-
PDO (PHP Data Objects)
MySQLi extension
PDO
-
PDO works on 12 different database systems.
-
If you change the database, you need to change the connection string.
-
It works with only MySQL database.
-
To change any other DB, need to rewrite entire code.
-
We will work with the MySQLi extension and MySQLi procedural
-
For Linux and Windows: The MySQLi extension is automatically installed in most cases, when php5 MySQL package is installed.
-
Here some predefined function for mysql database.
To Open a Connection
-
We will work with the MySQLi extension.
-
For Linux and Windows: The MySQLi extension is automatically installed in most cases, when php5 MySQL package is installed.
-
If you get error regarding password, execute this: ALTER USER 'yourusername'@'localhost' IDENTIFIED WITH mysql_native_password BY 'youpassword';

To Create a Table
-
Here we will create a table Employee, with five column: id, firstname, lastname, email & mobile no.
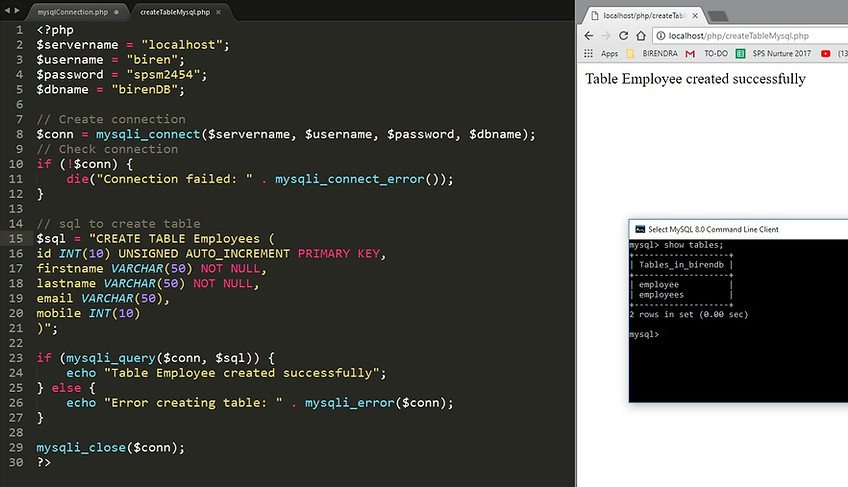
To Insert a Table
-
A prepared statement is a feature used to execute the same (or similar) SQL statements repeatedly with high efficiency.
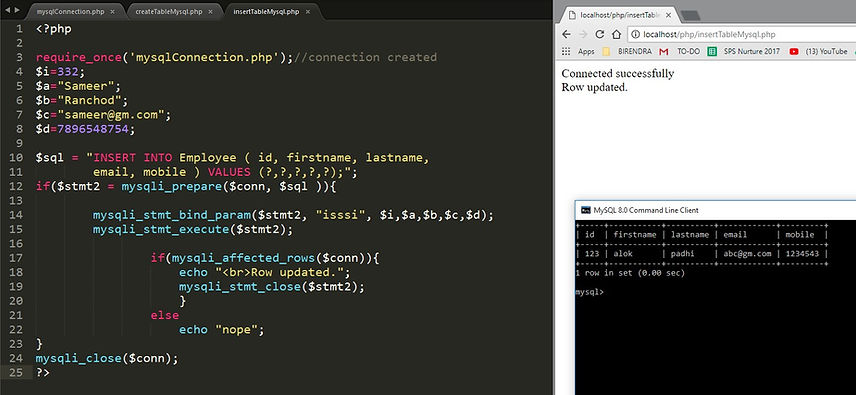
To Select a Table
-
The following example selects the id, firstname, last name from Employee table & displays it on the page.
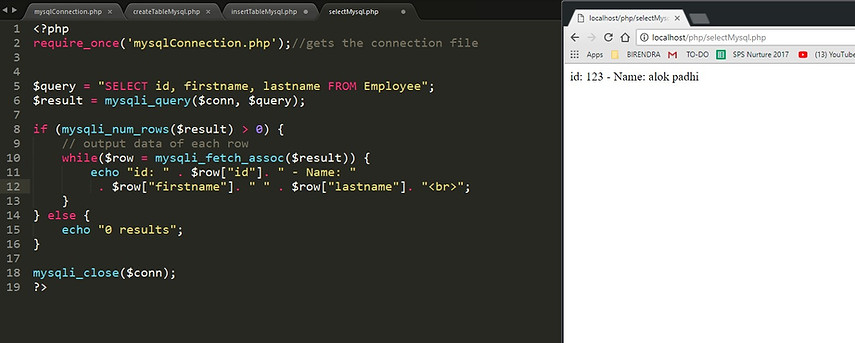
Reading from Form
-
PHP can read from a browser through a HTML form.
-
PHP $_POST['variable_name'] & $_GET('variable_name') holds the form data, are global variable.
-
The isset () function is used to check whether a variable is set or not.
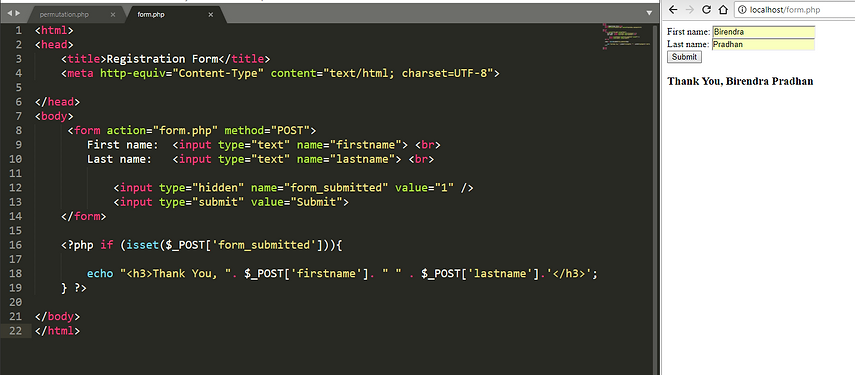
Program 2
-
Find the permutation of a string, "ab".
-
Ans. ab, ba
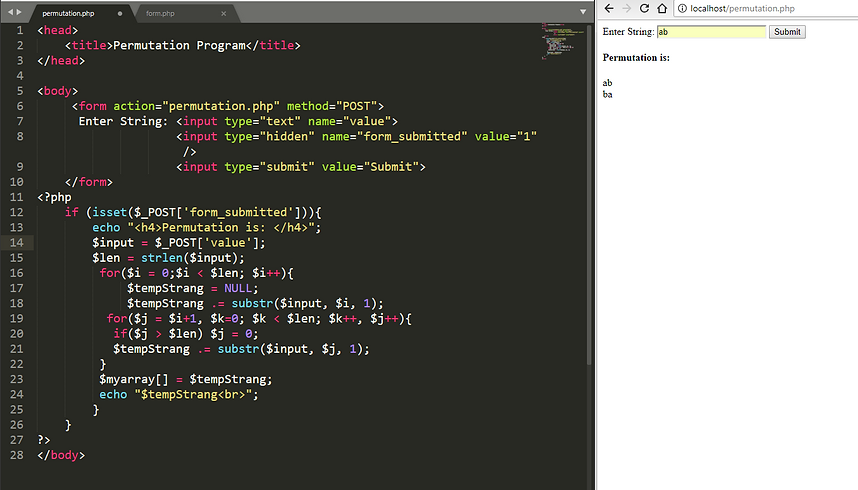
Program 3
-
Find Digit Identification if Sting have any number.
-
Input: Stone123Profits
-
Output: True
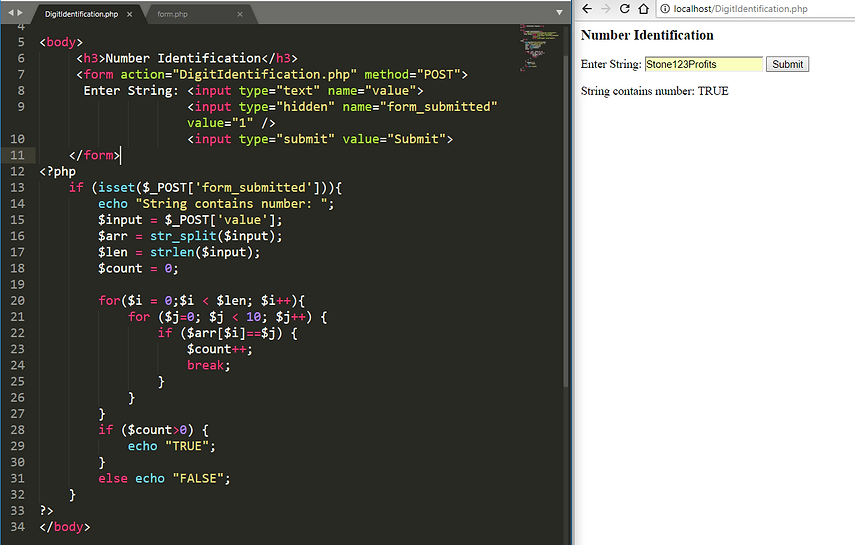
Program 4
-
Find & replace the 1st highest repeated character with user given character.
-
Input: acbabcabc, replace with: z
-
Output: zvczbczbc
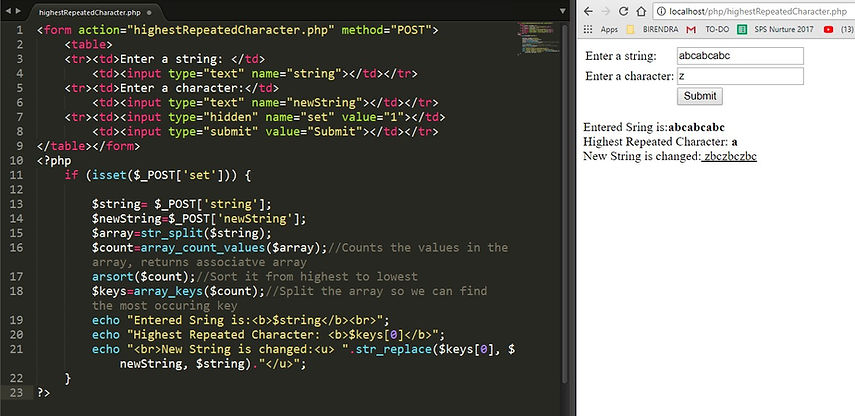
Program 5
-
WAP to print a Numerical Diamond Pattern.
-
Input: 5
-
Output: Prints diamond pattern
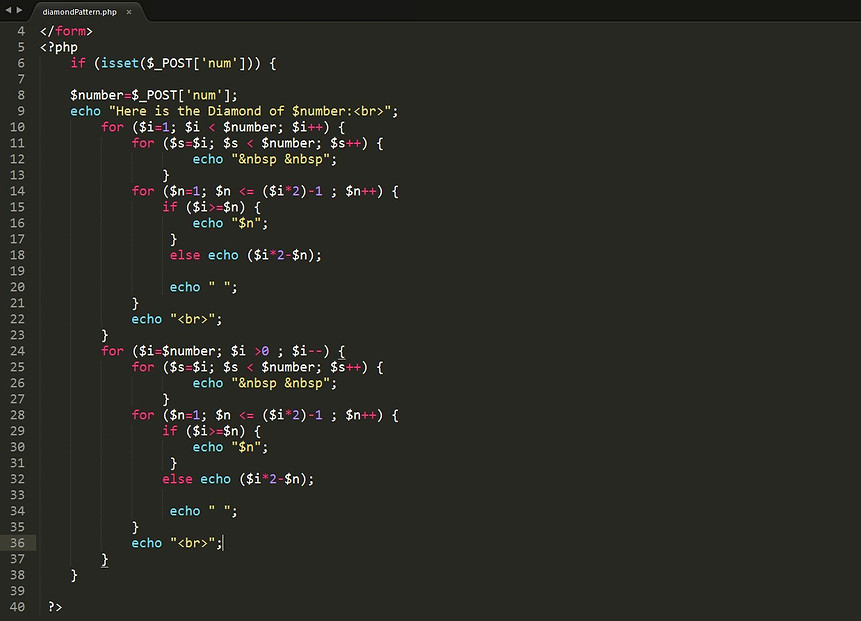
For upper pyrimid
For reverse pyrimid
-
Here is the output Diamond Pattern on the browser.
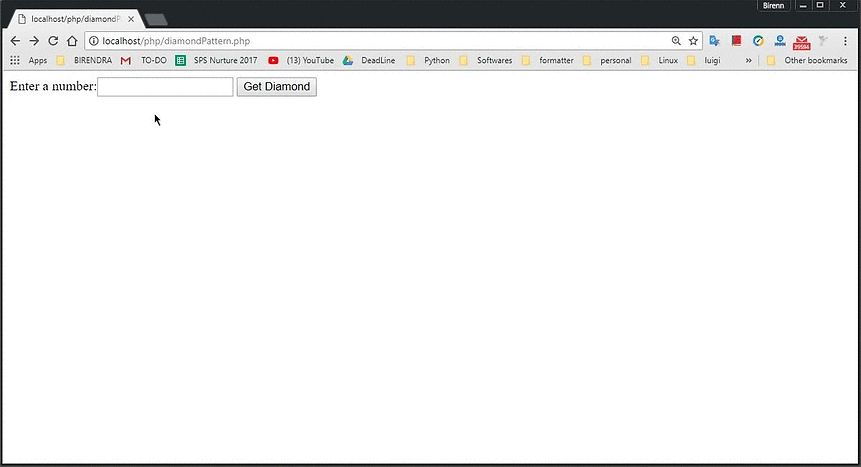
Program 6
-
Get the numbers from a String & Sum those numbers.
-
Input: Stone123Profits7
-
Output: 123 + 7 = 130
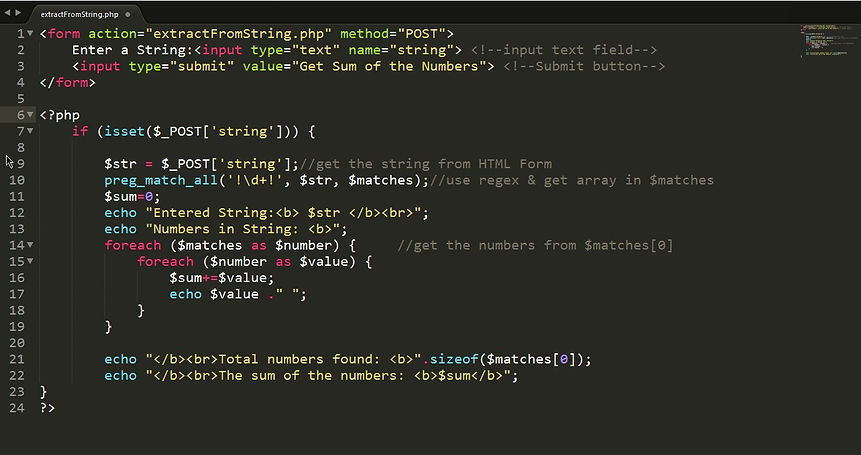
-
On the browser, the output is here.
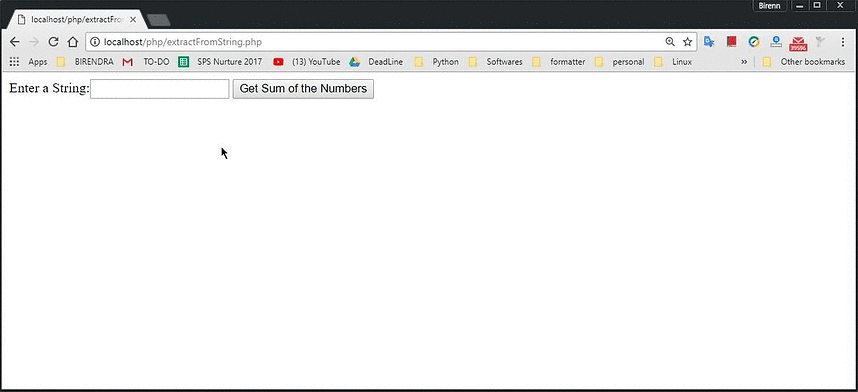
Program 7
-
Inter data into Database & retrive form database.
-
Input: Stone123Profits7
-
Output: 123 + 7 = 130
Exercise 2
-
Cont the number of occurrences of a substring in a full String.
-
Input: fullsring- abcdabegabchj , subsring- abc
-
Output: 2 times
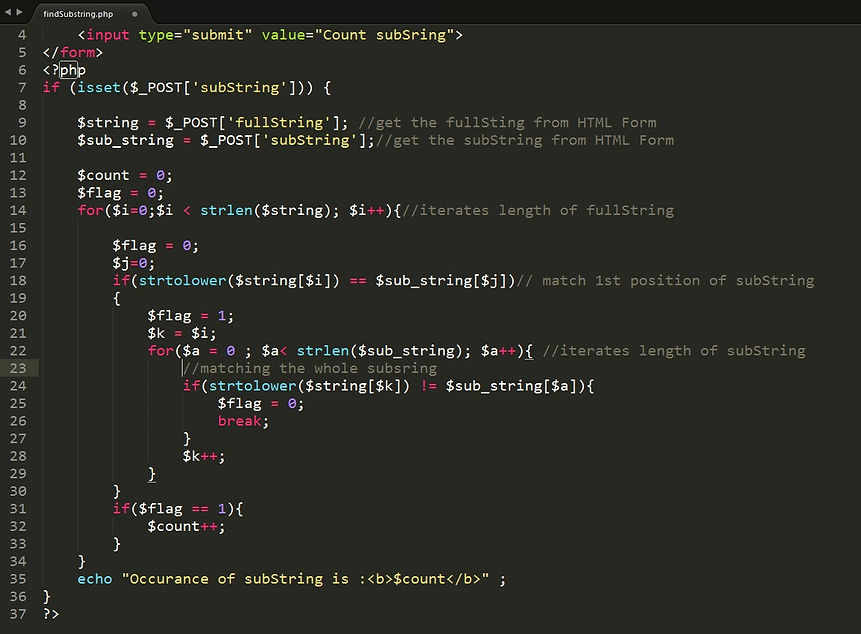
-
Here is the output on the browser.
-
It will search for a substring & count its occurrences on a full string.
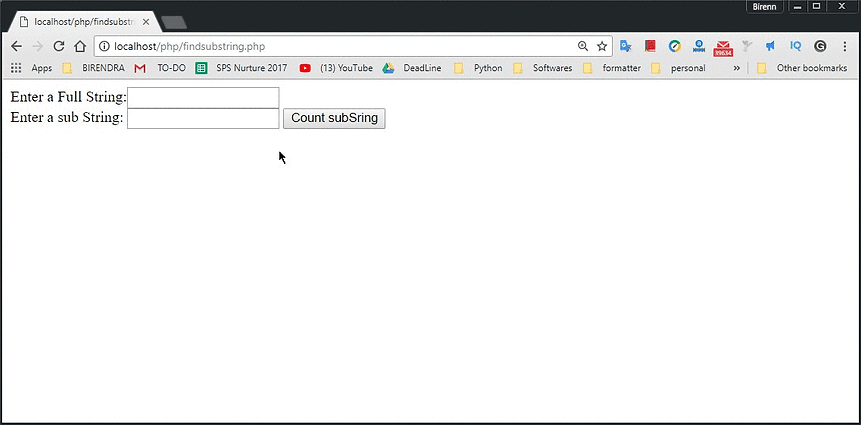
Exercise 3
-
Ask username & his age, then write a sentence.
-
Input: Ravi, 18
-
Output: Ravi is 18 years old.
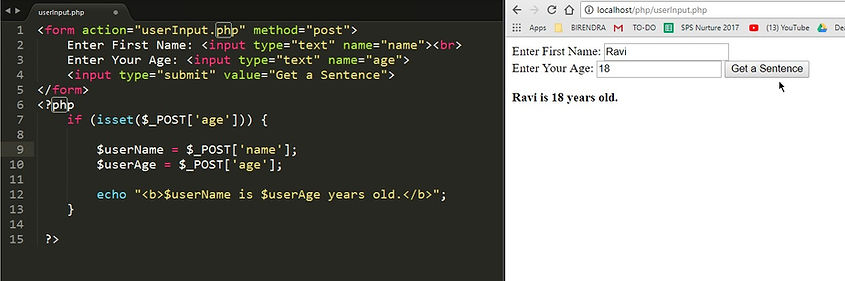
Exercise 4
-
Read from a file & write to a file. Read the 2nd & 4th line in a file.
-
Input:
-
Output:
Exercise 5
-
Count the number of occurrences of "Ravi" & replace with "Chandu".
-
Input:
-
Output:
Exercise 6
-
Draw Line, Circle, Rectangle & Star.
-
Input: N/A
-
Output: Geometric Shapes
-
To work with image file & shapes in PHP, we need to add GD library.
-
To configure this setting, go to C:\\php\php.in
-
Open php.in file > find gd2 > chage the extension value to php_gd2.dll
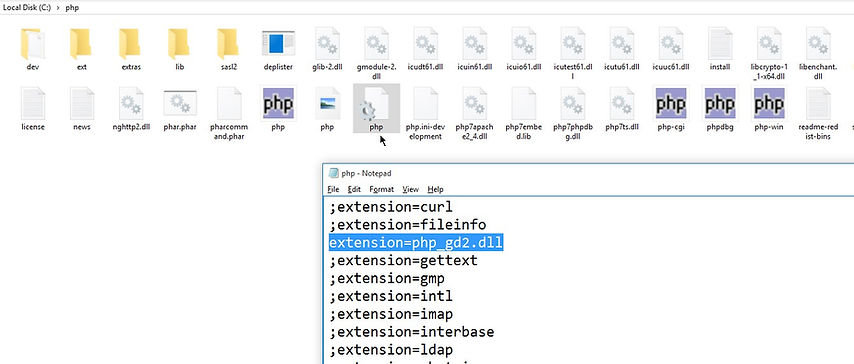
add php_gd2.dll
& remove ":"
-
We use some image function from PHP GD library.
No. of planes
R G B
Point 2 (x,y)
Point 1 (x,y)

-
The shapes are showing in the browser.
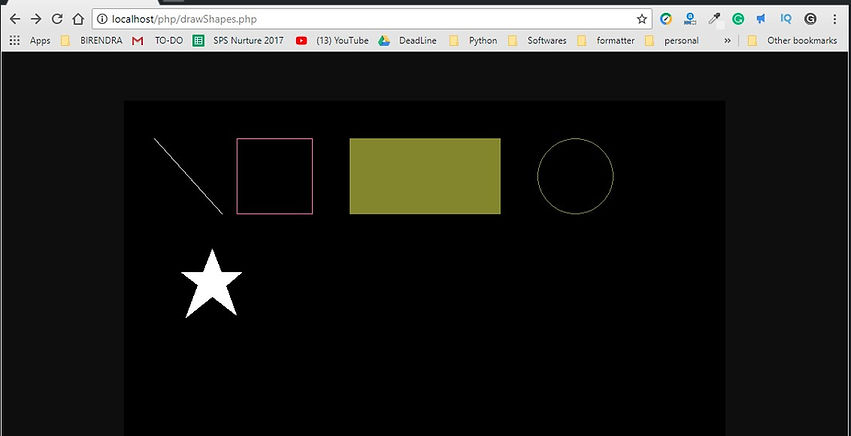
Exercise 7
-
WAP to write an excel data to the database.
-
Input: excel file
-
Output: Data into a database
bottom of page