C++
What is C++?
-
Compiled, general-purpose, free-form programming language.
-
A middle-level language, as it comprises a combination of both high-level and low-level language features.
-
Supports the object-oriented programming

History
-
It was created in 1979.
-
Developed by Bjarne Stroustrup at Bell Labs.
Why C++
-
Very fast.
-
Commonly used
-
Extension version of C language.
-
Object-oriented concept.
-
Exception handling.
C++ Installation
-
To run a C++ program we need a Text Editor & Compiler.
-
Or an IDE (Text editor + Compiler + more).
Compiler
-
Download C++ compiler.
-
We installed GCC compiler from MinGW.
Set Environment Variable Path
-
Set the bin folder of the compiler as Environment variable path.
Hello World
-
We wrote the Hello World program in C++.
-
But we build the environment for the Sublime Text editor for C++.
-
By pressing Ctrl + B, we got the result.

Basic Syntax
-
There is some base syntax we need to follow to write any C++ program.
Header file:
-
At the beginning of the program, we need to include a header file.
-
Here we use the iostream file, which provides input & output stream
Using namespace std:
-
It tells the compiler to use a namespace/library which contains some function.
-
If we did not mention using keyword, we can use the as follwing: std::cout << "Hello"
main():
-
It is the main function, which holds the execution part.
-
Return type is int.
cout():
-
It is a function for print on console.
Comment line
-
For single line comment: //
-
For multiple line comment: /* */
Variable & Datatypes
-
A variable holds a value, is the memory location by the compiler.
-
Variables can be declared anywhere in the program in C++ but must be declared before they are used
-
Each variable declaration has a datatype.
-
Here below example shows some datatypes with declaration & initialization.

cin & cout
-
std namespace has some console functions.
-
cin >> used to take user input on a console.
-
cout << used to print a string on a console.
Program 1
-
Ask username & age, print a sentence with those variables.
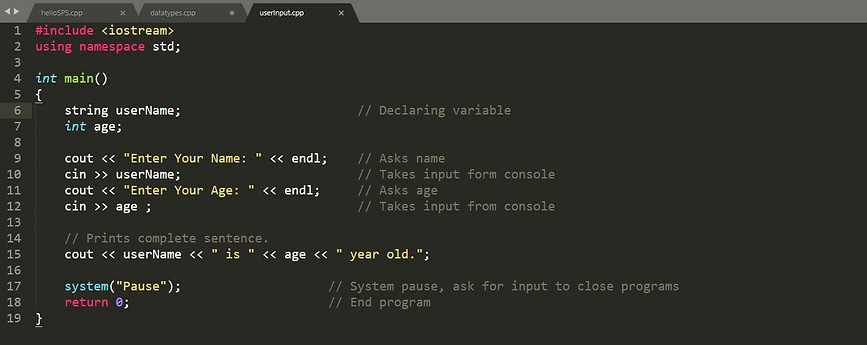
-
The output of the above program on the command prompt.

Compile .cpp file with gcc command
Run the exe file
Output
Class in C++
-
A class provides the blueprints for objects.
-
So basically an object is created from a class.
-
The public data members of objects of a class can be accessed using the direct member access operator (.).
-
A class definition starts with the keyword class.
-
Here is an example of class:

File Handling
-
In C++, we use fstream library for reading and writing a file.
-
There are 3 datatypes for file:
-
ofstream: For output file
-
ifstream: For input file
-
fstream: For both input and output
-
Header files <iostream> and <fstream> must be included in your C++ source file.
-
Functions for file functions are below:
-
outfile.open("file.dat", ios::out | ios::trunc ); - For open a file
-
void close(); - For close a file.
-
<< - For write to file.
-
>> - For read from file.
Write a File
-
We use an ofstream or fstream object instead of the cout object.
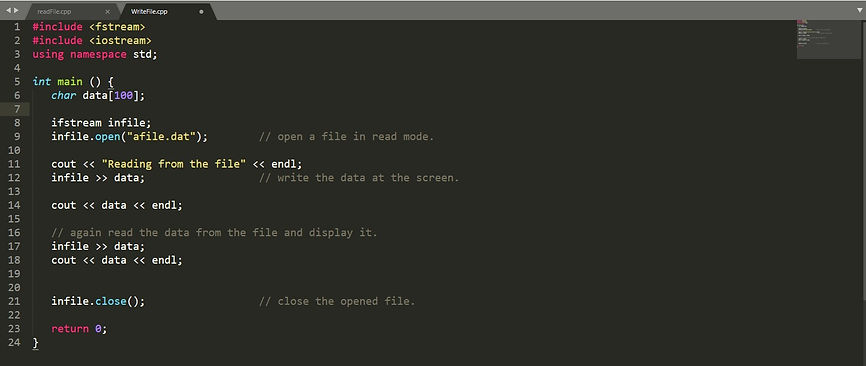
Read a File
-
We use ifstream or fstream object instead of the cin object.

Programs
Program 1
-
Finding the first non-repeated character in String.
-
Input: stonepfofits
-
Output: n

-
Output on Command Prompt:
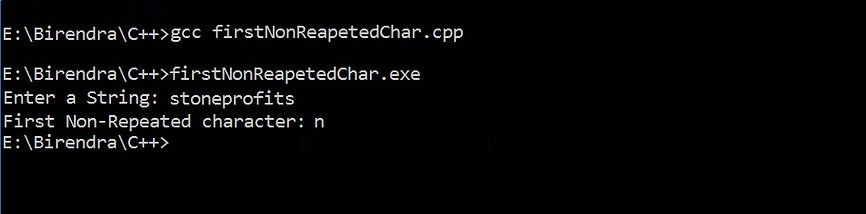
To Compile
To Execute
Program 2
-
Find the Permutation of string.
-
Input: ab
-
Output: ab, ba
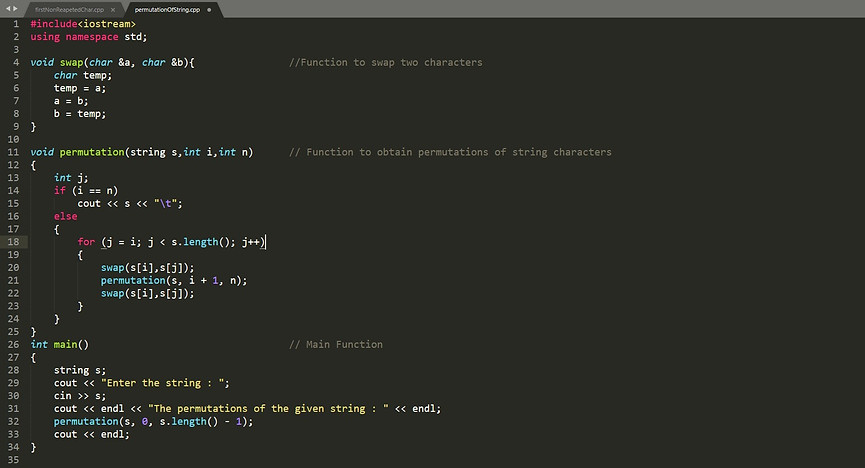

To Execute
User Input
Output
Program 3
-
Find Digit Identification if the string has any number.
-
Input: Stone123Profits
-
Output: True, if the string contains a number; False, if the string contains a number
Program 4
-
Find and Replace the 1st highest repeated character with user given character.
-
Input: abcabab
-
Output: pbcpbpb
Program 5
-
Write a program to print Diamond pattern.
-
Input: 5
-
Output: pattern
Program 6
-
Write a program to sum the numbers in a string.
-
Input: stone123profits7
-
Output: 130