C Language
What is C ?
-
Is a general-purpose computer programming language.
-
A High-level language.
-
It is the most widely used computer.
-
Mother of all modern languages.
History
-
It was created in 1972.
-
Developed by Dennis Ritchie.
Why C
-
Produce efficient programs.
-
Can be compiled on a variety of computer platforms.
-
Structured language.
Environment Setup
-
By downloading any IDE for C program comes with build in the environment.
-
Here, to set up a C program environment, we need to have 2 software:
-
Text Editor (Sublime Text 3)
-
C Compiler (GNU Compiler)
-
Compiler
-
A compiler translates programing language into executable code.
-
Programming languages may be any high-level language (eg. C, C++, Java, C#, Python)
-
Here we will have C compiler for executing .c file.
High-level Language
#include <stdio.h>
int main()
{
print ("Hello!/n")
}
demo.c
Machine-level Language
Compiler
0101010101011000101000010101010010101001001001001
demo.exe
-
To install GCC compiler, go to MinGW.org > download & install the compiler.
-
Make the bin directory as PATH for the PC.
The Hello World
-
Here is the Hello World program of C program with the minimum code as extension filename.c

-
Open the command prompt > go to the .c file directory
-
Type gcc helloSPS.c to compile.
-
It will generate the a.exe file on the same directory.
-
Type a to execute the program on the screen.

To compile helloSPS.c file
To execute the a.exe file
Here is the output
.exe file
.c file
Compiler on Sublime Text
-
Instead of writing commands lime for executing C programs, we can make Sublime Text editor do itself.
-
On Sublime Text option > Tools > Build System > New Build Path > paste the Jason code for GCC compiler > save as C.
-
Now we can compile & execute a C program by hitting Ctrl + B .
-
Here are gif shows how we made it.
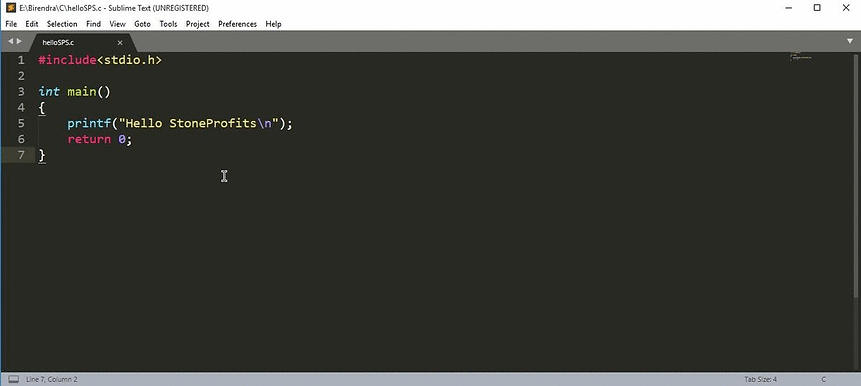
C Syntax
-
The above program has the basic syntax of C language
-
Following are the description:
-
#include <stdio.h>: it includes standard input outpur library function.( eg printf(), scanf() )
-
int main (): Every c programs has a main funcion to execute.
-
printf(): A funtion used to print on console.
-
return 0: Returns execution status to OS (0 or 1).
Variable in C
-
With variable we can store a data.
-
Variable stores on memory location.
-
One should declare a variable with datatype.
-
Here are some rules for declaring & defined variable with syntax.

Datatype
-
Datatype means the types of data that can store on a variable.
-
Eg. Integer, Floating, Sting etc.
-
In C, There are 4 types of a datatype.
Basic
int, char, float, double
Derived
Datatype
array, pointer, structure, union
Enumeration
enum
Void
non return type
Printf & Scanf
-
Both are the in-build function from stdio.h header file.
-
The printf() is used for output.
-
The scanf() is used for taking user input.
-
Both functions have a statement to print the which type of variable with a variable.
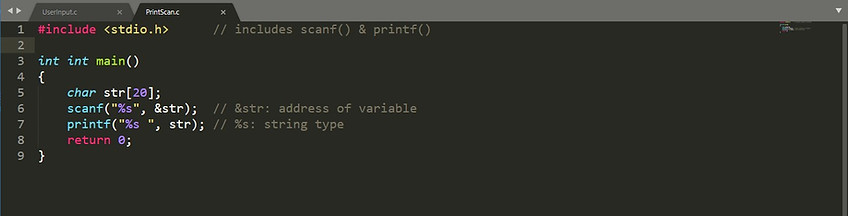
Format Specifier
-
Format specifier are used to take the input & output.
-
It says which type of data is in variable using scanf( ) & printf( )
Control Statement
If Else
-
The block of code will be executed according to a basic condition.
-
In C, there are statements available such as; if , if else, if else-if.
Do While
-
While loop is used to execute part of code multiple time untill condition is false.
-
The do-while loop is similar to While loop but at least execute once regardless of condtion.
For Loop
-
To iterate the code several time with condition and increment/decrement function.

Switch Case
-
Like if else-if ladder statement, the block of code from multiple conditions.
-
The switch expression and case value must be of integer or character type.
-
Every case should have break or goto keyword else it will follow fall-through condition.

Scope in Variable
-
Global Variable: Accessible throughout the program.
-
Local Variable: Accessible only inside of the program.
-
Formal Variable: While passing an argument with a function.
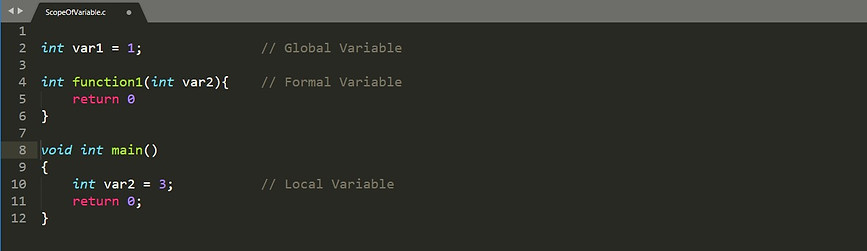
-
There are 4 storage classes used for defining the scope and lifetime of a variable.
-
These following keywords are used:
-
auto: Default storage class, applied to all local variables automatically.
-
register: Allocates memory in register than RAM, faster access than other variables.
-
extern: Visible to all the programs.
-
static: Once initialized, exists until the end of the program.

Pointer
-
Pointer stores the value of address of another variable & points toward it.
-
We need to declare a pointer before use.
-
Pointer datatype should be the same as the pointing variable.
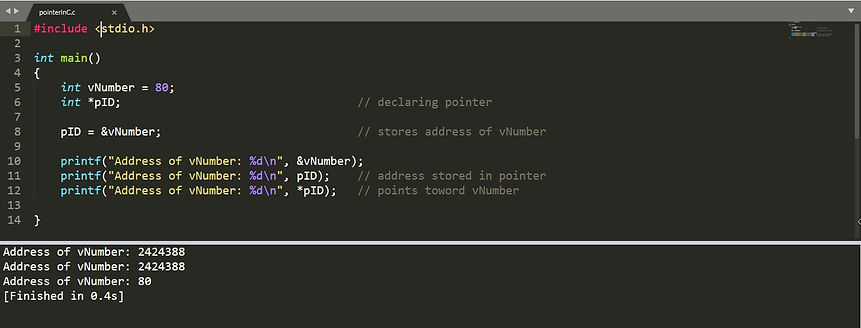
String
-
It is an array of character terminated by a null character.
-
We can declare a string with char keyword with or without array size.
-
Here is the 2 way of initializing the char.
-
char array
-
string literal

-
In C program, there is a lot of function for string manipulation.
-
Some of them are in below table.
Structure in C
-
Structure in C is a user-defined datatype.
-
Each element it stores is called a member.
-
Here, struct keyword is used to declare a structure.

File Handling
-
In C, there is some specific function to handle files on local disk.
-
The file may be of two types:
-
Text files ( .txt )
-
Binary files ( .bin )
-
To communicate with file and program, we need to declare a pointer of a datatype.
-
fopen() is a library function in stdio.h header file with file name & read/write mode.
-
To work with the file, there are some several steps we need to follow.
-
Creating a new file with a pointer
-
Opening a file
-
Reading/Writing to a file
-
Closing a file
Write a File
-
For reading a file, we use fprintf() & fwrite() functions.

Read a File
-
For reading a file, we use fscanf() & fread( ) functions.

Graphics in C
-
For drawing different shapes, fonts & colors, we use a graphics.h header file.
-
graphics.h contains lots of pre-defined functions to implement Graphical User Interface.
-
We can make graphics programs, animation & games.
-
Here is some functions from graphics.h header.
-
arc
-
bar
-
cricle
-
line
-
rectangle
-
ellipse
-
outtext
-
textwidth
-
Drawing in C
-
With graphics.h functions, we can draw circle, lines, rectangles, bars & other geometrical figures.
-
Below program is for drawing a some geometrical shapes.
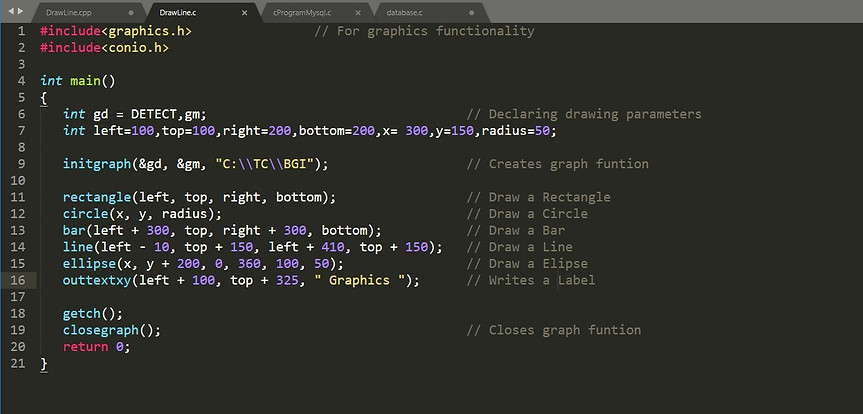
C with MySQL
-
There are several APIs available in internet allows us to interact with different databases
- We need to download the correspondence APIs which contains header file.
- And that header file should be kept in compiler's include folder to be accessed.
Incule mysql.h
-
Here we used mysql.h header file to interact MySQL database.
-
We downloaded & mysql.h and kept in complir's include folder.
-
Above picture shows where all the header path presents.
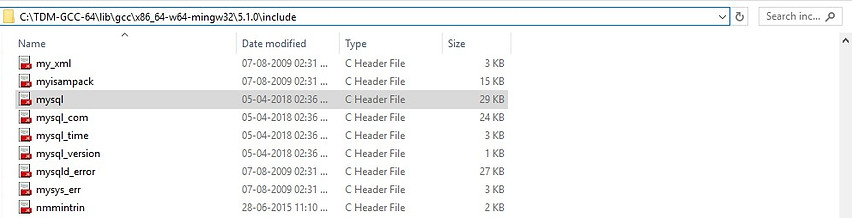
-
Download mysql.h file
-
keep in compiler's include folder
-
The above header file has lots of functions regarding MySQL.
-
Below program is the example of database connection.
-
The codes can be divided into 4 parts:
-
Initiation of a connection handle stucture
-
Creation of a connection
-
Execution of a query
-
Closing of connection
-
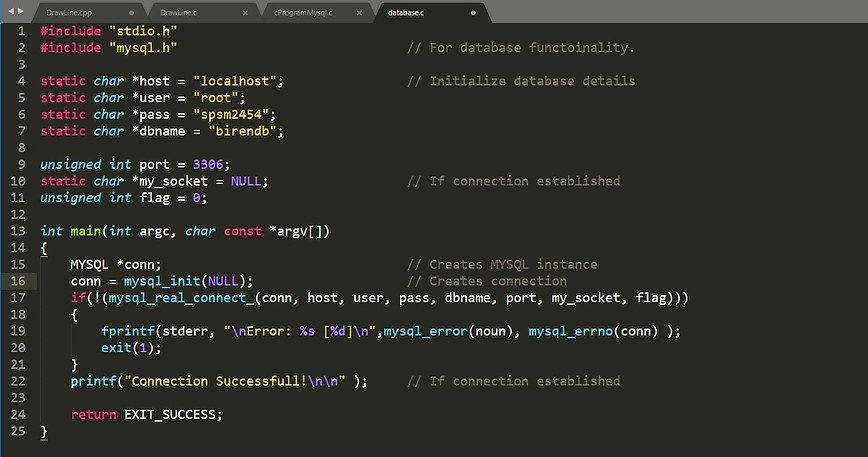
Program 1
-
WAP to ask username & his age, then write a sentence.
-
Input: Ravi
-
Output: 18
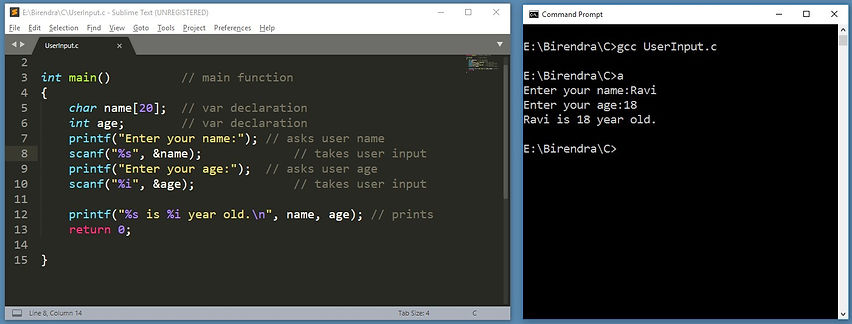
Program 2
-
Find the Permutation of string.
-
Input: ab
-
Output: ab, ba

Program 3
-
Find Digit Identification if the string has any number.
-
Input: Stone123Profits
-
Output: True, if the string contains a number; False, if the string contains a number
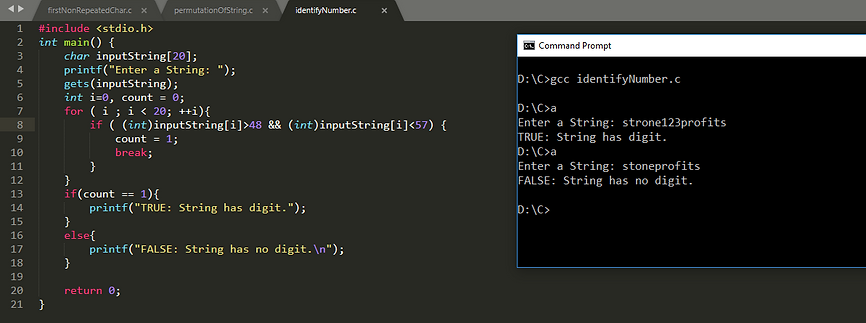
Program 4
-
Find and Replace the 1st highest repeated character with user given character.
-
Input: abcabab
-
Output: pbcpbpb

-
The out put of above program asks the user to enter a String & a character to replace with the highest repeated character.
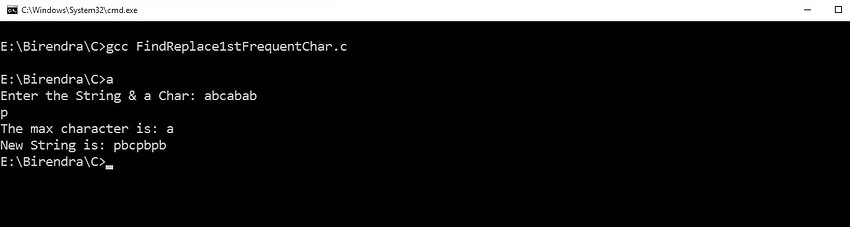
Compiles the program
Executing the program
Asks user to enter a String
Asks user to enter a Char
OUTPUT
Program 5
-
Write a program to print Diamond pattern.
-
Input: 5
-
Output: pattern
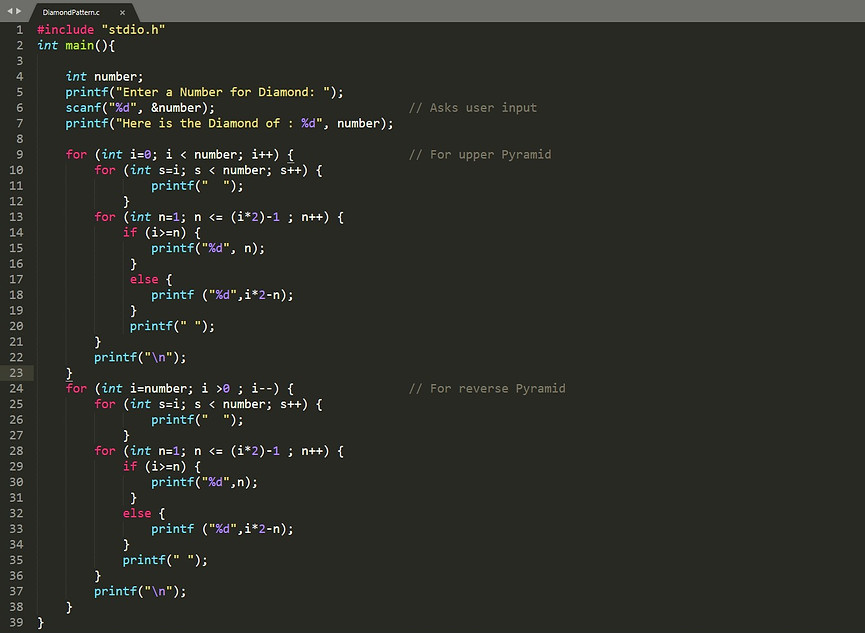
-
The above program prints the given diamond pattern on command prompt.

Compiles .c file
OUTPUT
Type a for executing above program
Program 6
-
Write a program to sum the numbers in a string.
-
Input: stone123profits7
-
Output: 130

-
In command prompt, we compile & executed the FindSumNumbersFromString.c file.
-
We used gcc command for compiling & a for executing the c file.
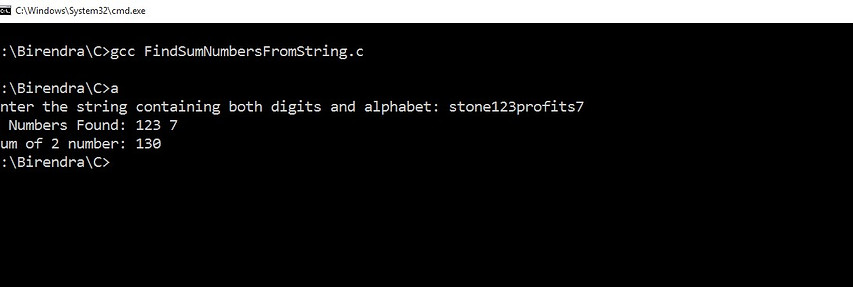
Compils .c file
OUTPUT
Ask user to input