Python
What is it?
-
Python is a programming language.
-
It is an interpreted language which executes line by line.
-
It is used for web development (server-side), software development, mathematics, system scripting.
-
Used on a server to create web applications and can connect to database systems.
-
It can also read and modify files.

History
-
It was created in 1991.
-
Designed by Guido van Rossum. (worked in Google & Dropbox)
Uses
-
Automatic Task
-
Make a game
-
Real-world problems
-
Server-client web development.
-
General purpose software.
-
Embedded software.
Why
-
Python was designed to for readability.
-
Python works on different platforms.
-
Python can be treated in a procedural way, an object-orientated way or a functional way.
-
One line code for swapping values; x,y = y,x
Cross-compilers to other languages
-
There are several compilers to run the python program on different platforms.
-
Here are some examples:
-
Jython compiles into Java bytecode, and execute on Java Virtual Machine.
-
IronPython is to run Python programs on the .NET Common Language Runtime.
-
Pyjs compiles Python to JavaScript.
-
Cython compiles Python to C and C++.
Python Installation
-
To check if you have python installed on a Windows PC:
-
Run the following on the Command Line (cmd.exe), python --version
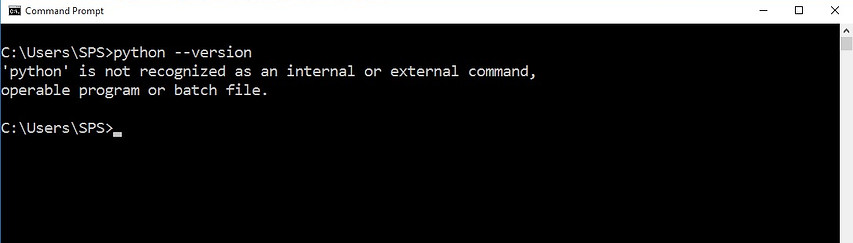
There is no Python installed on the PC.
Type python - -version
-
We can download it for free from the following website: https://www.python.org/
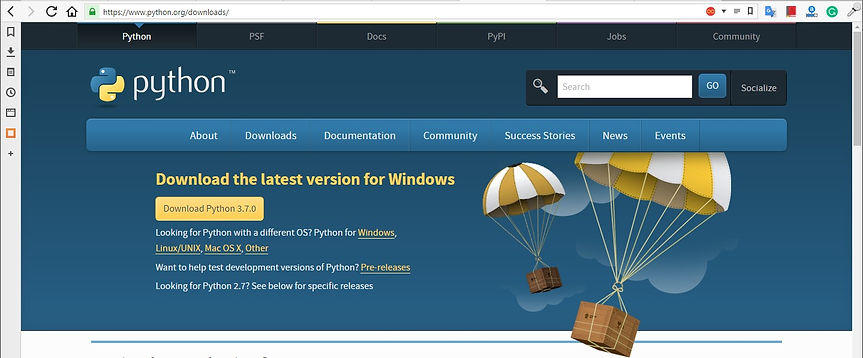
-
Open the .exe installer file.
-
Make sure you tick the "Add Python 3. to PATH" checkbox and click on "Install Now",
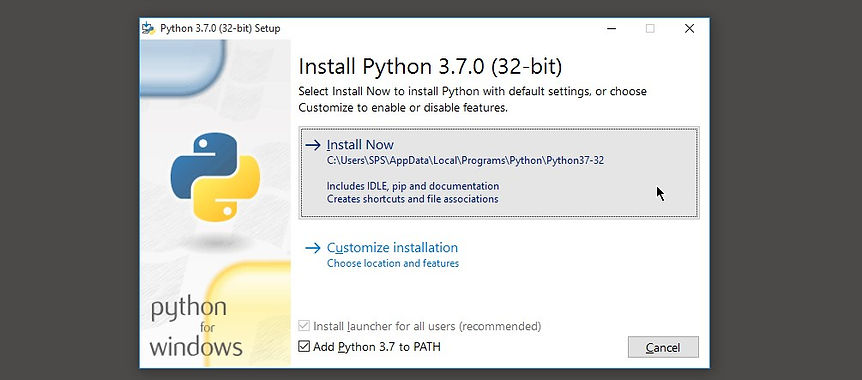
-
Verify the installation was successful by opening a command prompt and running the python command.
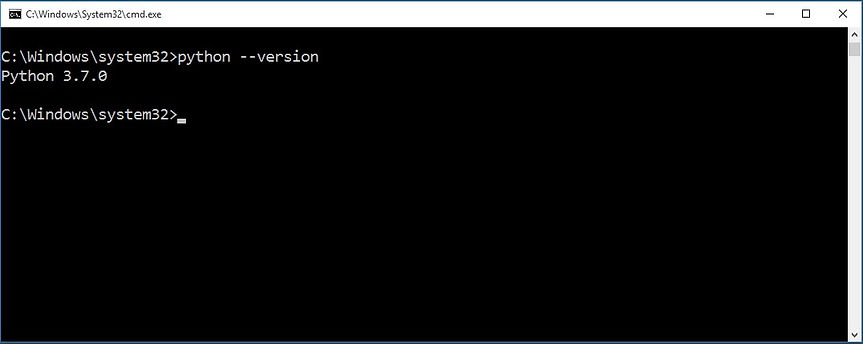
Type python - -version
Python installed on the PC.
The Hello World
-
Python is an interpreted programming language.
-
We need to write Python (.py) files in a text editor and then put those files into the python interpreter to be executed.
-
Where HelloWorld.py is the name of your python file.
-
We wrote the one line program in a text editor.
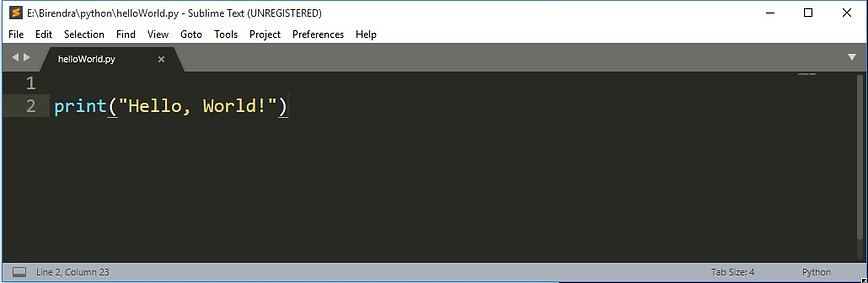
-
Save the python file, navigate the path on the command line.
-
To run the file, type python helloworld.py
-
The the print line shows here.

To run the python file
Here is the output.
Python Command Line
-
After running the Python command, the prompt changed to >>>
-
For a quick test, we can type commands (code) into the Python prompt results in answers in Python.
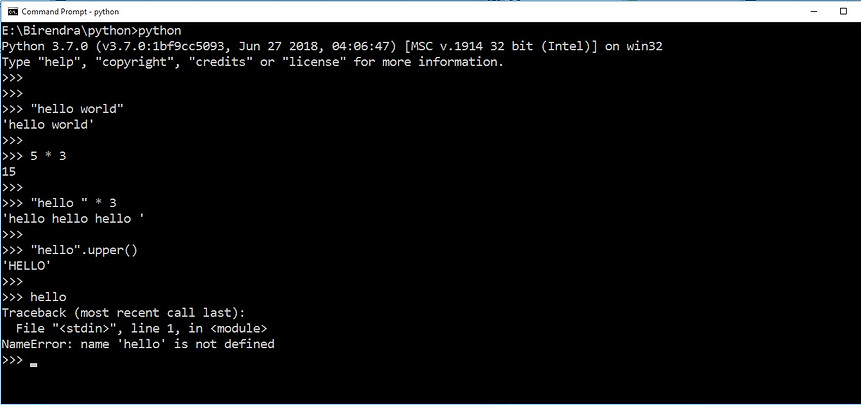
To pring the string
Operator
Operator & String
Python function
We got our first error
Python Command Line
Setting up Python with Sublime
-
To write the python program with Sublime Text 3 editor and make the editor compatible with python codes, here are some steps:
-
On Sublime Text, go to Tools > Command Pallets > type Install-Package Controller > click to install.
-
Type and install SublimeREPL or Python 3.
-
This plug-in lets the user to an interactive interpreter for several languages.

Install the Python 3 plug-in
-
For command line output in the editor, go to Tools > Built System > select Python.
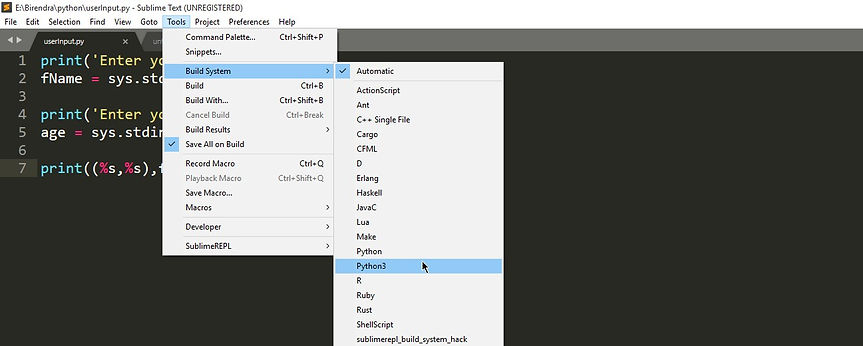
-
Hit Ctrl + B or Tool menu > Build for command line output.
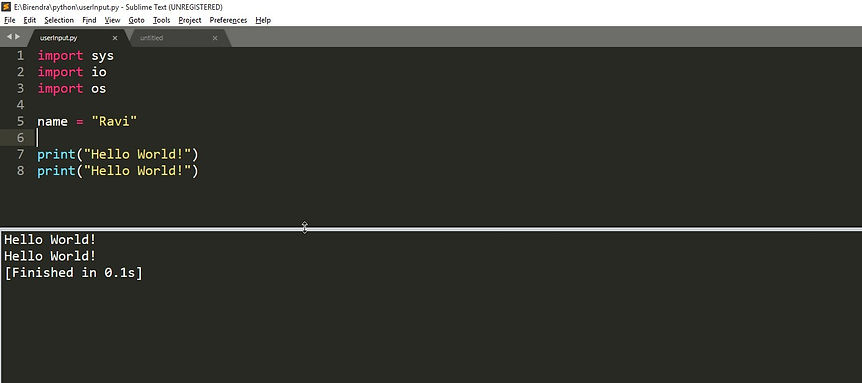
Coding window
Command line wondow
(output)
Python Syntax
Indentations in Python
-
Python uses indentation to indicate a block of codes.
-
It gives an error if no indent in a block.
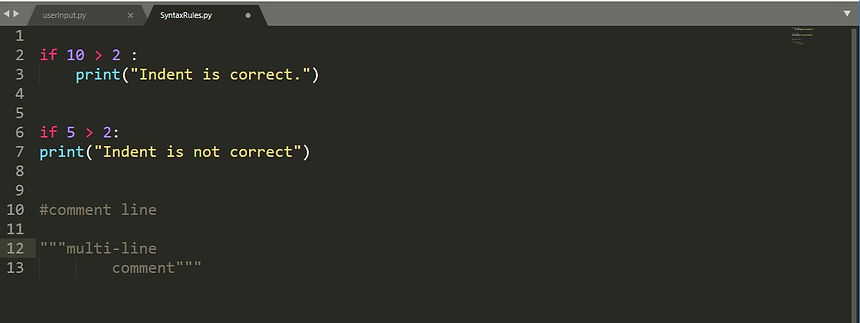
Indentation
No-Indentation
Comments


Variable Declaration
-
Python has no command for declaring a variable.
-
The variable is being declared when we assign a value.
-
The right side of the = operator is being assigned to a variable.
-
The variable type keeps changing after re-assign.
-
Varible name should start with alphabet or underscore & can contain numbers, alphabets or underscore.
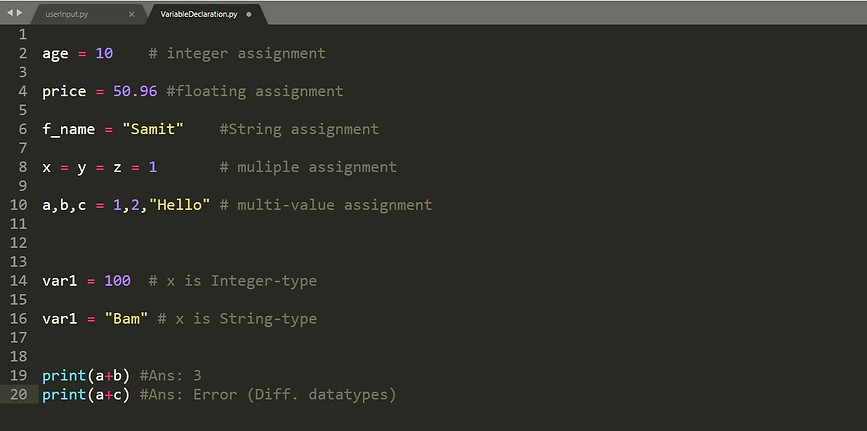
Variable declaration
Datatypes
-
The data stored in memory can be any types.
-
To verify the type of an object in Python, use the type( ) function:
-
Here are some Python datatypes.
Datatypes
Number
stores number
String
"char"
List
['a','b','c']
Tuple
('a', 'b', 'c')
Dictionary
{'key' : 'value'}
value can't change
Integer
100
Float
100.12
Complex
5j, 3=4j
Number
-
Number objects are created when you assign a value.
String:
-
Stores number of character.
-
In Python, 'hello' is the same as "hello".
-
Subsets of strings can be taken using the slice operator ([ ] and [ : ] ) with index.
List [ ]
-
Lists are the most versatile of Python's compound data types. (multiple datatypes)
-
A list contains items separated by commas and enclosed within square brackets [ ].
Tuple ( )
-
Unlike List, the value in a tuple cannot be changed.
-
It is a read-only List.
-
It is enclosed within parentheses.
Dictionary { }
-
Dictionary is a kind of hash table type.
-
It is a collection which is unordered, changeable and indexed.
-
It is also possible to use the dict() constructor to make a dictionary.
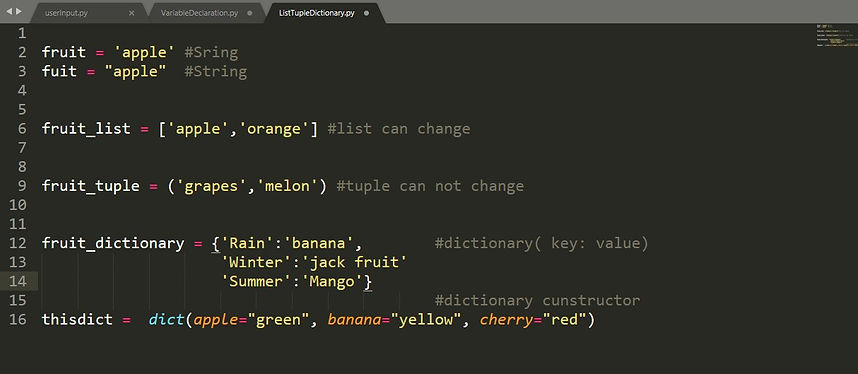
-
There is some function for accessing & manipulating the string, tuple, list, and dictionary.
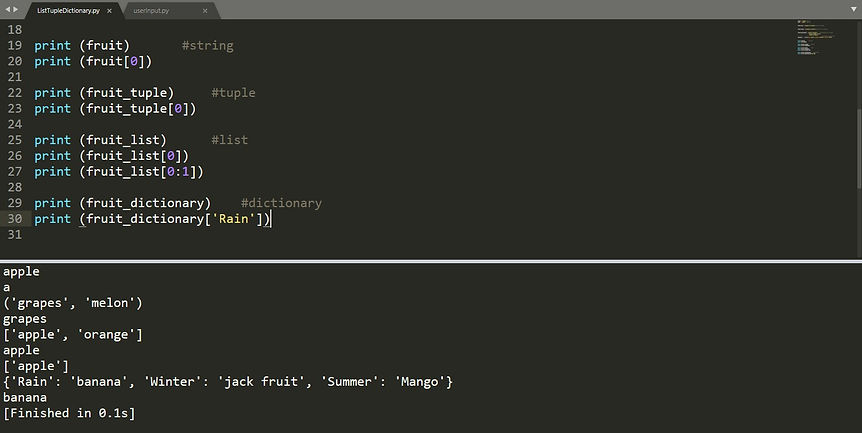
Type Casting
-
We can specify a type on to a declared variable.
-
Casting in python are done using some below functions:
Operator
-
Operators are used to perform some operation to a variable.
-
Similar to another program, Python has different types of operators as follows:
-
Arithmetic operators ( + - * / % ** // )
-
Assignment operator ( = += -= %= >>= |= ^= )
-
Comparison operator ( == != < > >= <= )
-
Logical operator ( and or not )
-
Identity operators ( is is not )
-
Membership operators ( in not in )
-
Bitwise operator ( & | ^ ~ << >> )
Conditional Statement
-
Python supports comparison operator to make a condition.
-
Thes conditions can be used in a loop and if condition.
-
On condition we need to use indentation for the code.
if statement
-
if the keyword is used to execute some code in a specific condition.
if elif statement
-
elif keyword is used in python, if the previous conditions were not true, then do this condition.
if else statement
-
If all the previous conditions were false, then perform else statement.
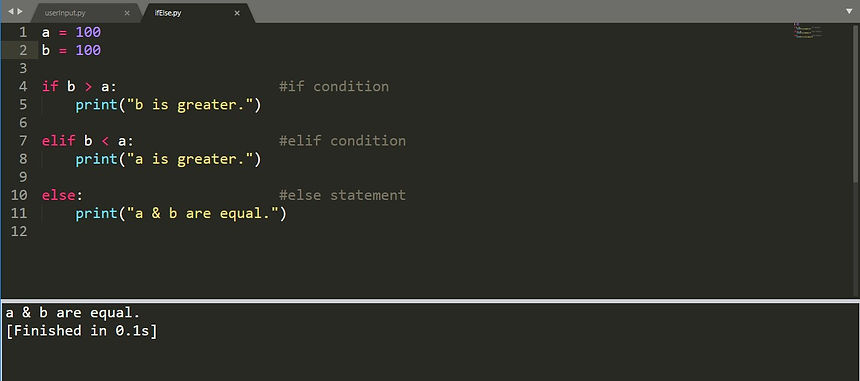
Loop control statement
-
For iteration, there are 2 loop in python:
-
For Loop: Iterates the statement for a limited time, when the condition is true.
-
While Loop: The statements are being executed unless the given condition is false.
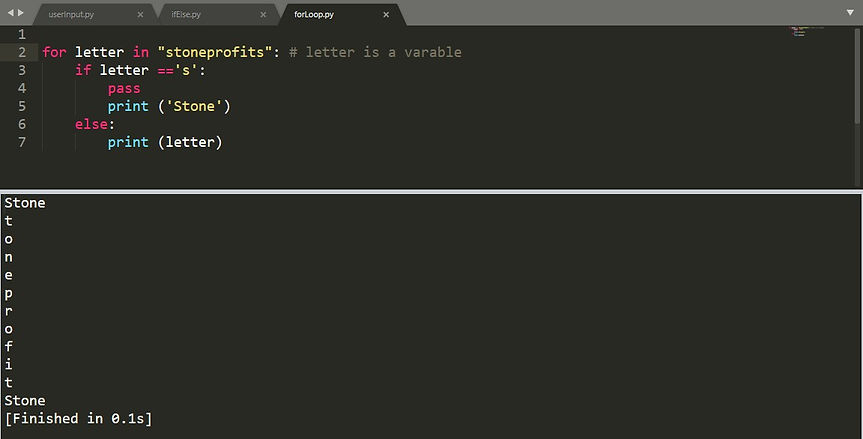
// For loop
-
In Python, else statement can be associated with while and for loop.
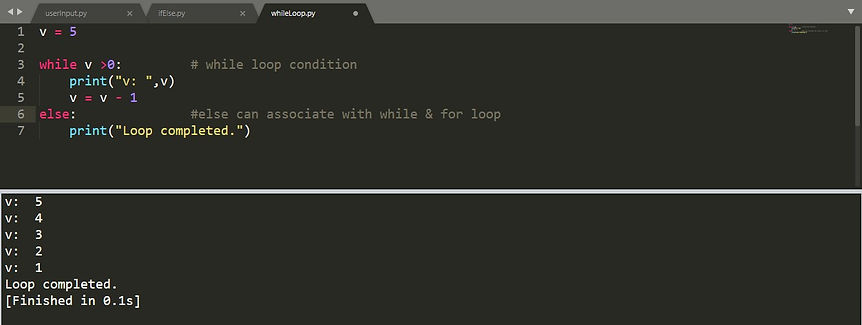
// While loop
// While - else
Loop control statement
-
Inside a loop, python has 3 statement to control the loop:
-
pass: When we have no statement to pass.
-
continue: To skip the rest of the body of the loop, and continue iterating.
-
break: Terminates the loop statement and transfers execution to the statement immediately.

// continue
// break
1st loop
2nd loop
Programing Concept
function
-
A Function is a block of code runs only when it is called.
-
We can pass parameter (value or reference).
-
The function block starts with def keyword followed by the function name and parentheses( ).
-
And the function block indented after column :
-
The function can return a value.
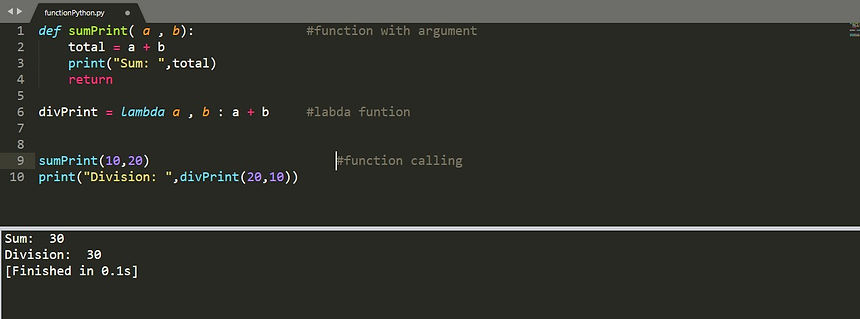
module
-
Like a library, a module is a file (.py) consisting of Python code; classes, functions & variable.
-
We can use that module in our application by import keyword followed by module name.
-
While python interpreter gets import statement it looks for the corresponding module file here:​
-
Current path
-
Shell variable path
-
Default path: C:\Users\SPS\AppData\Local\Programs\Python
Built-in Module
-
Python comes with some built-in modules. (eg. sys, io, platform)
-
For easy work, we can import those.

User-defined Module
-
A user can make a module by having the required variable, function and classes.
-
Save the file as .py extension, and keep in the current path.
-
And use those functions, value form that python file.
Python Package Manager
-
Python has a Package manager for downloading different the modules.
-
The later version of Python 3.4 has installed pip.
-
Here how to install a package:
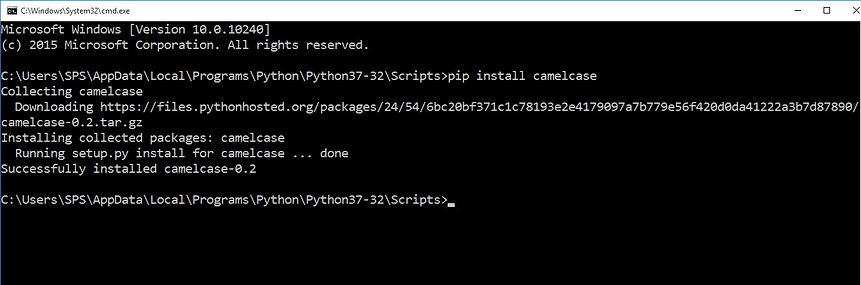
To download & install camelcase module
-
Once the package is installed we can use that.
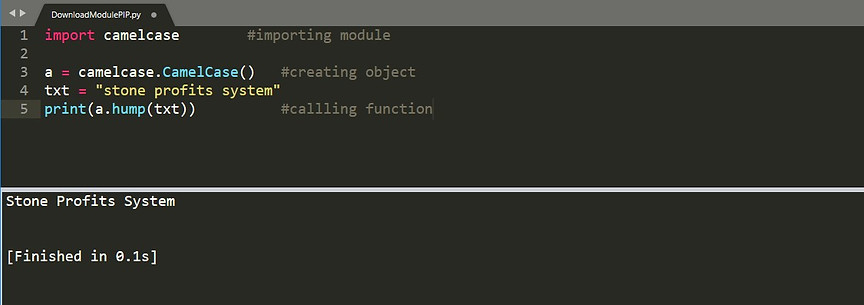
File I/O Handling
-
Python provides 2 functions to get the user input.​
-
raw_input ( )
-
input( )
-
We can create, read, update & delete the files using some functions.
-
The open( filename, mode ) function takes two parameters; filename, and mode.
-
r : Read
-
a : Append
-
w : Write
-
x : Create
-
For handing mode of file:
-
t: Text mode
-
b: Binary mode
Write File
-
Here is an example of writing a file.
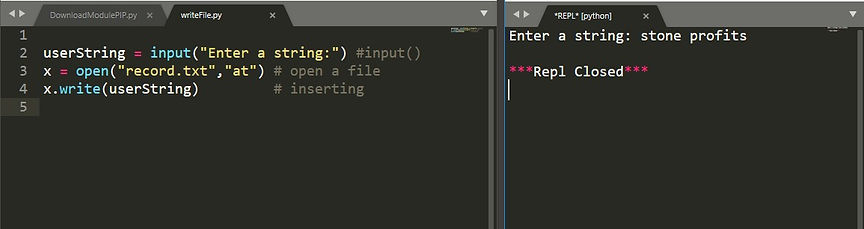
-
The text file gets affected as below.
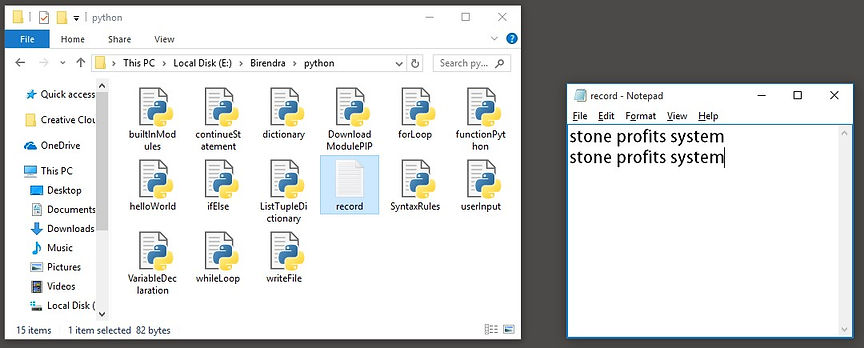
Read File
-
In python, open( ) function returns a file object, where it has a read( ) function is used to read the content.​
-
Here is the example for reading the text file data as string.
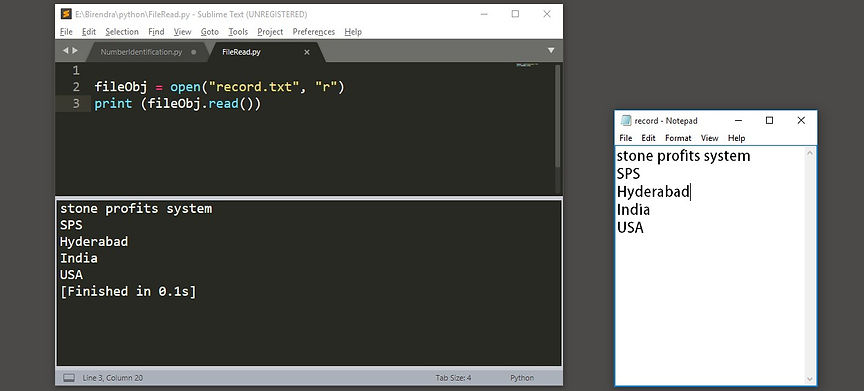
Exersice: Read the 2nd and 4th line of the file.
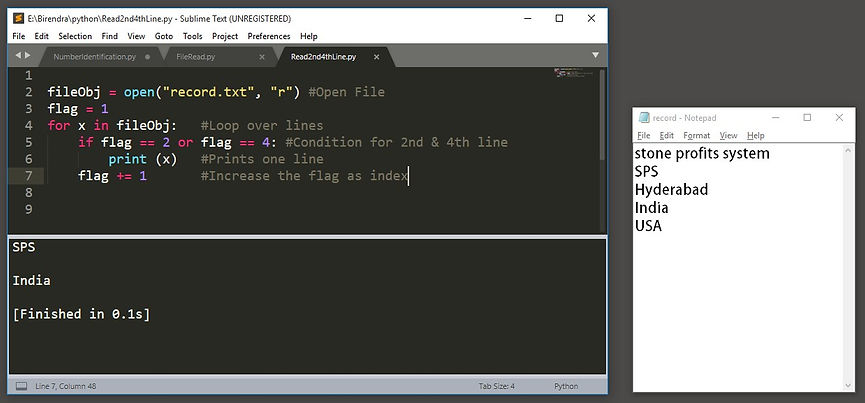
OS Module
-
The is os module which allows us to remove the file and rename the file as well.
-
ps.remove("note.txt") : Remove a file in local directory.
-
os. rename("old.txt","new.txt") : Rename a file
-
os.mkdir("Folder1") : Make a new folder.
-
os.getchwd( ) : Get the current working directory.
-
os.chdir("/Folder/SPS/qPlus") : To chage the current working directory.
-

Python & MySQL
-
We can use Python in a database application.
-
Here we have MySQL database installed to work with.
-
We can choose any database for our application.
MySQL Driver
-
In order to work with a MySQL database in Python, we need to install a driver for respective database.
-
MySQL Connector is the driver we are going to intall on PIP.
-
We can download and install the driver from the internet.
-
Open the PIP path in CMD > type python -m pip install mysql -connector
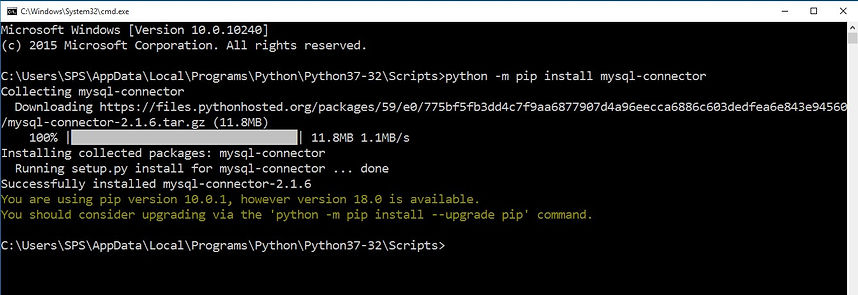
Download & Install the mysql connector on pip
Create Connection
-
After installing the driver, we can create a connection,
-
We need to import module, create a connection object with a database credential.
-
A short program for testing the connection between Python and MySQL.
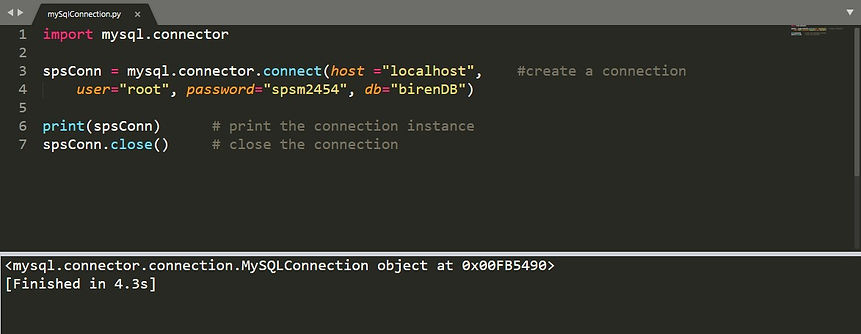
Connection established.
SELECT Table
-
To run any query on database, we need to create a cursor class object.
-
The cursor object allows us to run the query & handle the returned record.
-
In the MySQL database, we have the following data in a database.
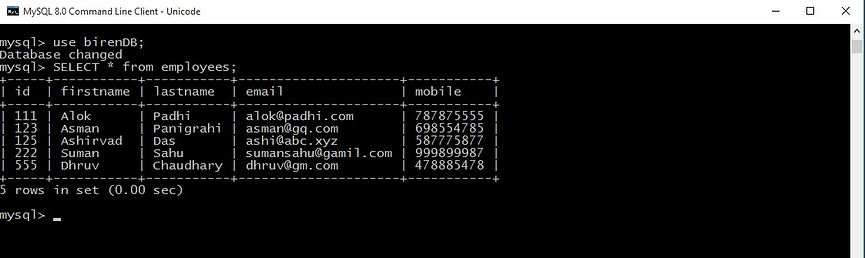
-
There are some functions to getting the record from the database on below.
-
cursor.fetchall ( ) - Returns all rows from executed records.
-
cursor.fetchone ( ) - Returns only one row.(first row)​​
-
-
Here is a program for SELECT query and getting the records.
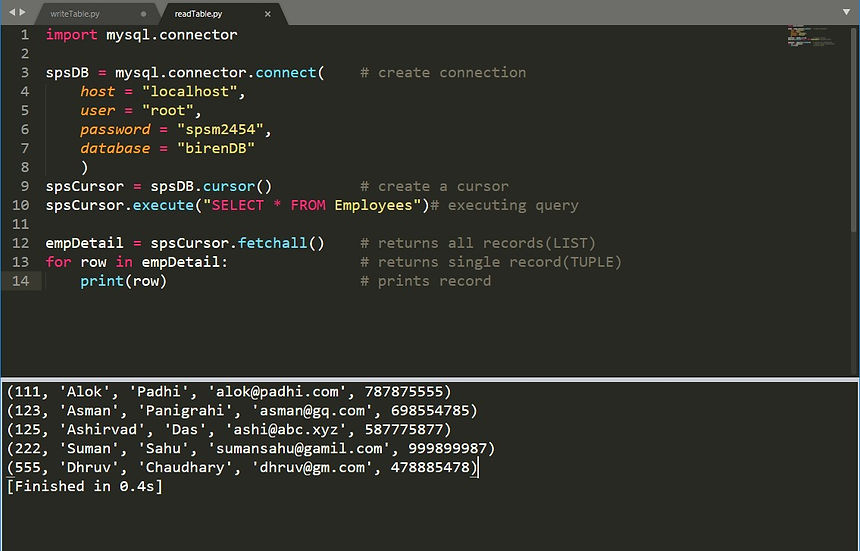
SELECT Table
INSERT Table
-
To insert the data from Python to MySQL can be done using by following steps.
-
First: Call the execute ( ) function with query & data as an argument.
-
Second: Call commit ( ) function for making changes on database.
-
For inserting to database functions are:
-
cursor.execute (sql query , data) - For inserting one-row
-
cursor.executemany (sql query, data_Tuple) - For inserting multiple rows.
-
dbConn.commit ( ) - For saving the chages.
-

INSERT Table
-
The GIF shows how it works after executing the above python program & checked on MySQL database.

Excel To Database
-
Here we are going to import excel files in Python and write those spreadsheet data into databse.
-
There are several modules available for work with a spreadsheet.​

Data
Excel

python
Data

mySQL Databse
-
In this case, we will download and install xlrd module.
-
To do so, open CMD > type and enter pip install xlrd

-
The below program is for importing a excel file from the local directory.
-
And inserting to MySQL database.

-
After running the python program, the 4 rows are been updated to database.

Python Graphics
-
For graphic work, there are several modules we can put on to work in python.
Python Turtle
-
Turtle is a python module for drawing comes with python package.
-
All we need to import the module, make an instance, draw a shape, and put on the screen.
-
Here is the following steps on below.
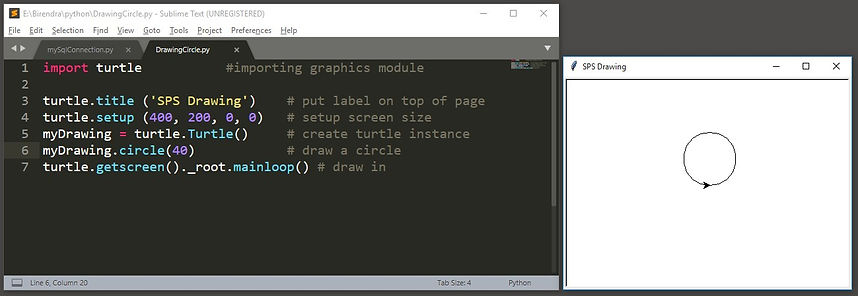
Turtle Function
-
Here are some turtle functions of turtle module for drawing.
Exercise
-
Draw a circle, line, rectangle & star.
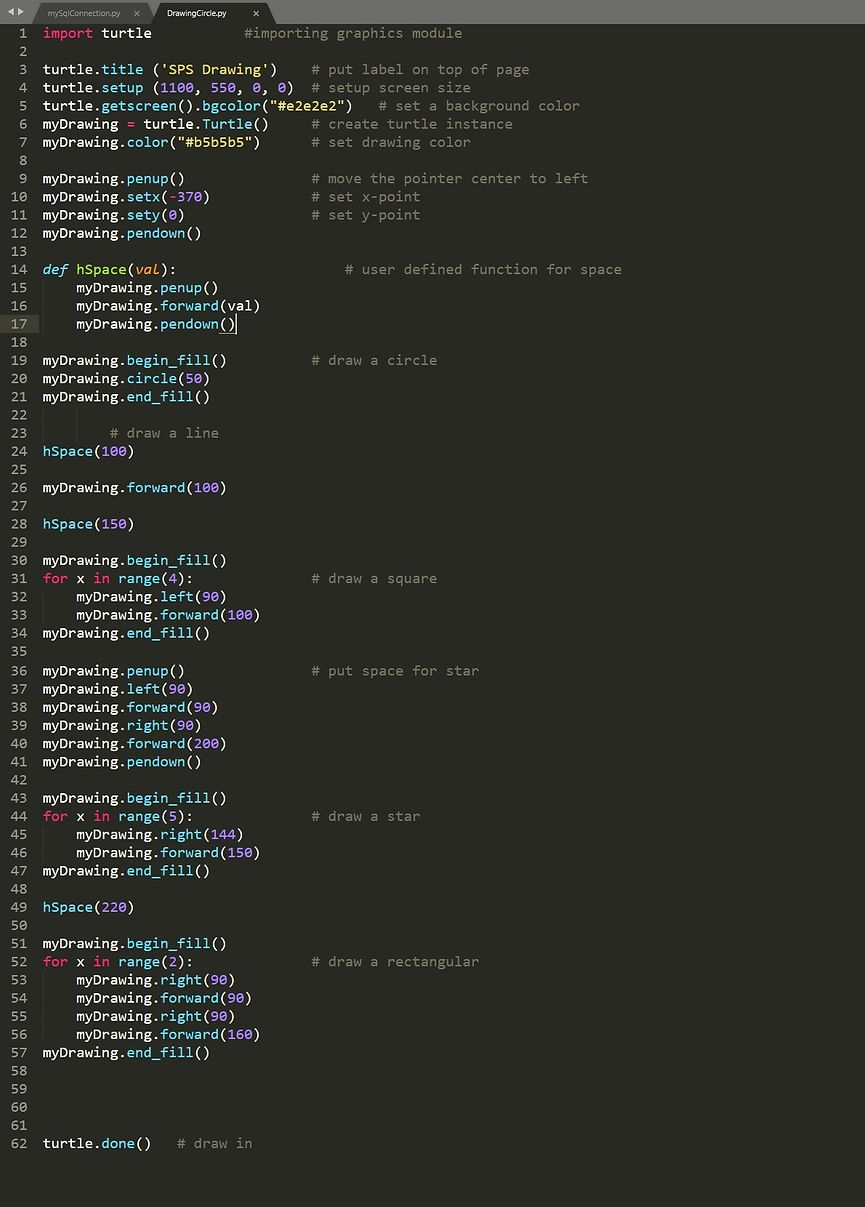
-
Output:
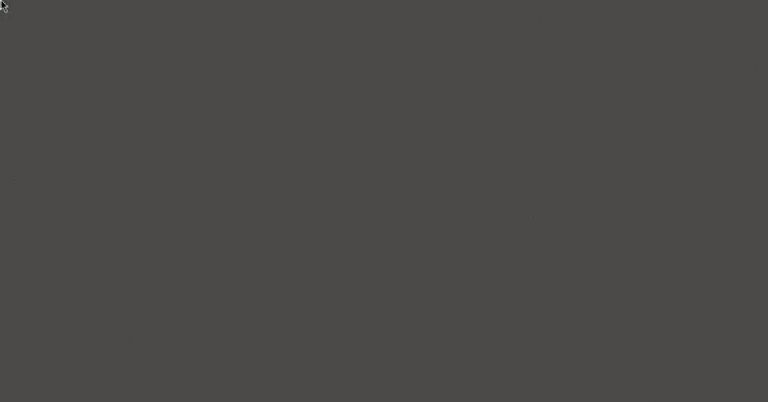
Programs
Program 1
-
Finding the first non-repeated character in String.
-
Input: stonepfofits
-
Output: n

Program 2
-
Find the Permutation of string.
-
Input: ab
-
Output: ab, ba
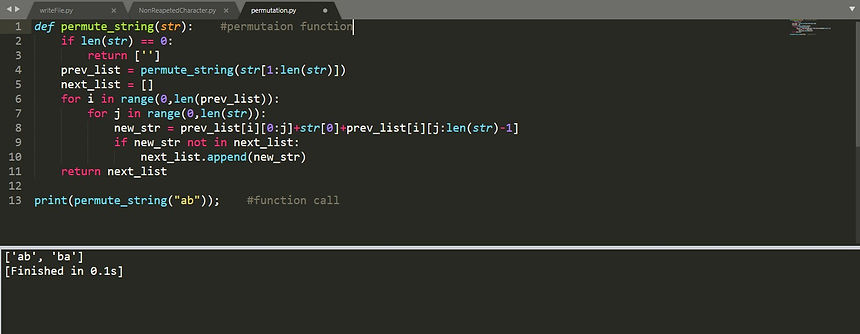
Program 3
-
Find Digit Identification if the string has any number.
-
Input: Stone123Profits
-
Output: True, if the string contains a number; False, if the string contains a number
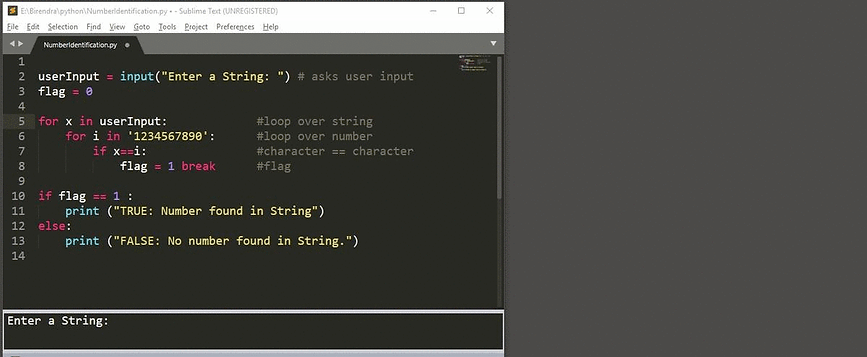
Program 4
-
Find and Replace the 1st highest repeated character with user given character.
-
Input: abcabab
-
Output: pbcpbpb

Program 5
-
Write a program to print Diamond pattern.
-
Input: 5
-
Output: pattern

Program 6
-
Write a program to sum the numbers in a string.
-
Input: stone123profits7
-
Output: 130
